Kelas ByteArrayInputStream ing paket java.io bisa digunakake kanggo maca array input (byte).
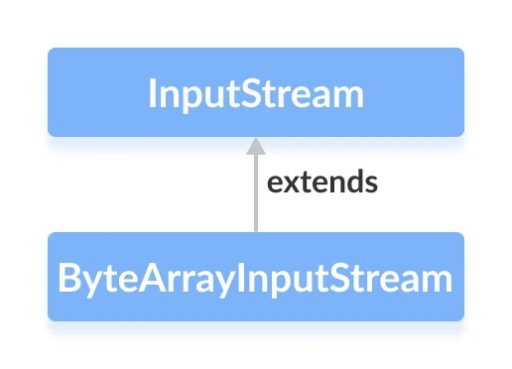
Kanggo nggawe stream input array byte, kita kudu ngimpor paket java.io.ByteArrayInputStream dhisik . Sawise ngimpor paket kasebut, kita duwe rong konstruktor sing kasedhiya kanggo nggawe aliran input:
ByteArrayInputStream input = new ByteArrayInputStream(arr);
ByteArrayInputStream input = new ByteArrayInputStream(arr, 2, 2);
Ana 4 lapangan ing kelas:
// Byte array provided by the creator of the stream
protected byte buf[];
// Index of the next character to read from the input stream's buffer
protected int pos;
// Current marked position in the stream
protected int mark = 0;
// Index is one greater than the last valid character in the input stream's buffer
protected int count;
Lan iki konstruktor kita:
public ByteArrayInputStream(byte buf[]) {
this.buf = buf;
this.pos = 0;
this.count = buf.length;
}
public ByteArrayInputStream(byte buf[], int offset, int length) {
this.buf = buf;
this.pos = offset;
this.count = Math.min(offset + length, buf.length);
this.mark = offset;
}
Metode kelas ByteArrayInputStream
Metode | Tumindak |
---|---|
int maca () | Maca bita sabanjure data saka stream input iki. |
int maca (byte b[], int mati, int len) | Maca sawetara bita saka stream input lan nyimpen ing buffer array b . mati minangka offset menyang target array b . len iku jumlah maksimum bita kanggo maca. |
long skip (long n) | Skips n bita input saka stream input iki. Ngasilake jumlah bita dilewati (bisa uga kurang saka n yen kita tekan mburi stream input). |
int kasedhiya () | Ngasilake jumlah bita sing isih bisa diwaca (utawa dilewati) saka aliran input iki. |
void reset() | Ngreset buffer menyang posisi sing ditandhani. Posisi ditandhani 0 kajaba posisi liyane ditandhani utawa offset beda kasebut ing konstruktor. |
tandha booleanSupported() | Priksa manawa InputStream iki ndhukung tandha/reset. Ngasilake bener kanggo ByteArrayInputStream . |
batal tutup() | Ora nindakake apa-apa. |
void mark(int readAheadLimit) | Nyetel ingtandhalapangan padha karo posisi saiki. Yen cara reset diarani, maca sabanjure bakal diwiwiti saka posisi kasebut. Parameter readAheadLimit ora digunakake lan ora mengaruhi prilaku metode kasebut. |
Ayo goleki kanthi luwih cetha babagan metode kasebut lan deleng kepiye cara kerjane.
maca ()
Yen sampeyan pengin maca bita saka ByteArrayInputStream kaya sampeyan saka InputStream biasa , sampeyan bisa nggunakake cara maca () .
public static void main(String[] args) {
byte[] array = {1, 2, 3, 4};
try (ByteArrayInputStream input = new ByteArrayInputStream(array)) {
for (int i = 0; i < array.length; i++) {
int data = input.read();
System.out.print(data + ", ");
}
} catch (IOException e) {
e.printStackTrace();
}
}
kasedhiya ()
Yen sampeyan pengin mriksa apa ana soko ing buffer Panjenengan, sampeyan bisa nelpon kasedhiya () cara.
public static void main(String[] args) {
byte[] array = {1, 2, 3, 4};
try (ByteArrayInputStream input = new ByteArrayInputStream(array)) {
System.out.println("Bytes available for reading: " + input.available());
input.read();
System.out.println("Bytes available for reading " + input.available());
input.read();
System.out.println("Bytes available for reading " + input.available());
} catch (IOException e) {
e.printStackTrace();
}
}
Kita bakal weruh sing nomer bita kasedhiya kanggo owah-owahan maca sawise saben maca saka buffer.
Output:
Byte kasedhiya kanggo diwaca: 3
Byte kasedhiya kanggo diwaca: 2
skip (long n)
Sampeyan bisa nggunakake cara skip () kanggo skip nomer tartamtu saka bita lan ora maca.
public static void main(String[] args) {
byte[] array = {1, 2, 3, 4};
try (ByteArrayInputStream input = new ByteArrayInputStream(array)) {
input.skip(2);
while (input.available() != 0) {
int data = input.read();
System.out.print(data + ", ");
}
} catch (IOException e) {
e.printStackTrace();
}
}
Output:
reset()
Cara iki ngreset posisi stream buffered menyang posisi ditandhani pungkasan. Iku posisi 0 kajaba tandha beda disetel.
public static void main(String[] args) {
byte[] buf = {65, 66, 67, 68, 69};
try (ByteArrayInputStream input = new ByteArrayInputStream(buf)) {
System.out.println("Read: " + input.read());
System.out.println("Read: " + input.read());
System.out.println("Read: " + input.read());
System.out.println("Read: " + input.read());
System.out.println("Calling reset() method");
input.reset();
System.out.println("Read: " + input.read());
System.out.println("Read: " + input.read());
} catch (IOException e) {
e.printStackTrace();
}
}
Kita bakal weruh sing nelpon reset () cara njupuk kita menyang titik wiwitan stream.
Output:
Waca: 66
Waca: 67
Waca: 68
Cara reset () Telpon
Waca: 65
Waca: 66
tandha (int readAheadLimit)
Metode tandha () kelas ByteArrayInputStream nyetel tandha internal ing posisi bait saiki, yaiku, sanalika sawise bait sing diwaca sadurunge. Cara iki njupuk parameter sing nuduhake jumlah bita sing bisa diwaca sawise tandha sadurunge stream dadi ora sah. Kanthi gawan, yen tandha ora disetel kanthi tegas, ByteArrayInputStream menehi tandha posisi 0 utawa posisi offset sing diterusake menyang konstruktor. Penting kanggo dicathet yen tandha readAheadLimit ora relevan kanggo kelas iki.
/* Note: For this class, {@code readAheadLimit}
* has no meaning.
*
* @since 1.1
*/
public void mark(int readAheadLimit) {
mark = pos;
}
Punika conto nyetel tandha ing ByteArrayInputStream nggunakake cara tandha () lan ngreset () . Kita bakal nambah telpon menyang metode tandha () kanggo conto sadurunge:
public static void main(String[] args) {
byte[] buf = {65, 66, 67, 68, 69};
try (ByteArrayInputStream input = new ByteArrayInputStream(buf)) {
System.out.println("Read: " + input.read());
System.out.println("Read: " + input.read());
System.out.println("Read: " + input.read());
input.mark(5);
System.out.println("Read: " + input.read());
System.out.println("Read: " + input.read());
System.out.println("Calling reset() method");
input.reset();
System.out.println("Read: " + input.read());
System.out.println("Read: " + input.read());
} catch (IOException e) {
e.printStackTrace();
}
}
Kita bisa ndeleng manawa posisi stream saiki wis diganti.
Output:
Waca: 66
Waca: 67
Waca: 68
Waca: 69
Metode reset () Telpon
Waca: 68
Waca: 69
markSupported()
Cara markSupported () ngijini sampeyan mriksa apa tandha bisa disetel. Kanggo mangerteni saka ngendi asale nilai bali, ayo pindhah menyang kode metode kasebut:
/**
* Tests if this {@code InputStream} supports mark/reset. The
* {@code markSupported} method of {@code ByteArrayInputStream}
* always returns {@code true}.
*
* @since 1.1
*/
public boolean markSupported() {
return true;
}
Cara kasebut mesthi ngasilake bener . Ayo nyoba iki ing laku.
public static void main(String[] args) {
byte[] buf = {65, 66, 67, 68, 69};
try (ByteArrayInputStream bais = new ByteArrayInputStream(buf)) {
boolean isMarkSupported = bais.markSupported();
System.out.println("isMarkSupported: " + isMarkSupported);
System.out.println("Read: " + bais.read());
System.out.println("Read: " + bais.read());
bais.mark(1);
System.out.println("Read: " + bais.read());
isMarkSupported = bais.markSupported();
System.out.println("isMarkSupported: " + isMarkSupported);
bais.reset();
isMarkSupported = bais.markSupported();
System.out.println("isMarkSupported: " + isMarkSupported);
} catch (IOException e) {
e.printStackTrace();
}
}
Sawise nglakokake metode tandha () lan ngreset () , stream kita tansah siap lan ndhukung tandha:
Output:
Waca: 65
Waca: 66
Waca: 67
isMarkSupported: bener
isMarkSupported: bener
cedhak()
Lan kanggo ngerti cara sing cedhak , ayo ndelok ing jero:
/**
* Closing a {@code ByteArrayInputStream} has no effect. The methods in
* this class can be called after the stream has been closed without
* generating an {@code IOException}.
*/
public void close() throws IOException {
}
Dokumentasi kanggo metode cedhak ngandhani yen nutup ByteArrayInputStream ora ana pengaruhe. Metode kelas ByteArrayInputStream bisa diarani sawise stream ditutup tanpa mbuwang IOException .
Apa sing bisa kita simpulake?
Kita butuh ByteArrayInputStream nalika pengin maca data saka array byte. Biasane nggawe pangertèn kanggo nggunakake kelas iki ing kombinasi karo kode liyane sing ngerti carane bisa karo InputStreams tinimbang dhewe.
GO TO FULL VERSION