程序执行过程中可能会出现错误。对于每个程序员来说,从初学者到真正的专业人士,这都是一个常见问题。并非所有错误都是由开发人员的过错引起的。其中一些很难预测,有时甚至是不可能的。例如,在下载程序时,网络连接可能会突然断开或断电。这种情况称为例外。Try 和 catch 是用于处理异常的构造部分。
Java try 和 catch 块
当发生错误时,Java 通常会停止并生成错误消息。这个过程称为“Java抛出异常”。Java 提供了处理异常的特殊工具。其中之一是 try...catch...finally 构建。这是 try 块、catch 块和finally 块的语法。//try block
try {
// Block of code to try
}
//try catch
catch(Exception e) {
// Block of code to handle errors
}
finally {
// Optional block of code
}
当 try 块中发生异常时,控制权将传递给 catch 块,后者可以处理异常。如果没有找到这样的块,则会向用户显示未处理的异常消息,并停止程序的进一步执行。为了防止这种紧急停止,您需要使用 try..catch 块。 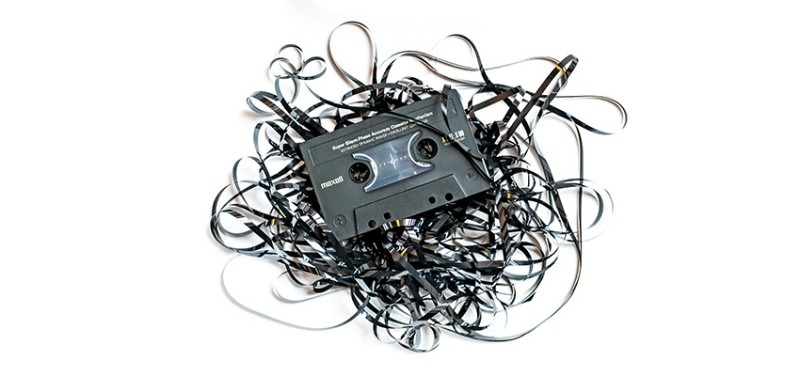
简单介绍一下try、catch、finally、 throws关键字
Java 中的异常处理基于在程序中使用以下关键字:- try——定义可能发生异常的代码块;
- catch — 定义处理异常的代码块;
- finally — 定义一个可选的代码块,但如果存在,则无论 try 块的结果如何,都会执行。
- throw——用于引发异常;
- throws——用在方法签名中,警告方法可能抛出异常。
try-catch 构造的简单示例
假设我们有一个带有一些数组的程序。public class TryTest {
public static void main(String[] args) {
int[] myArray = new int[5];
myArray[7] = 8;
System.out.println(myArray[7]);
}
}
由于我们尝试访问索引不存在的数组元素,因此程序将退出并出现错误:
线程“main”中出现异常 java.lang.ArrayIndexOutOfBoundsException:索引 7 超出长度 5 天的范围。TryTest.main(TryTest.java:6) 进程已完成,退出代码为 1
让我们修改这个程序并使用 try-catch 处理这个异常。首先是 try 块,然后是 catch 块。
//try catch example
public class TryTest {
public static void main(String[] args) {
try {
int[] myArray = new int[5];
myArray[7] = 8;
System.out.println(myArray[7]);
} catch (Exception myEx) {
System.out.println("The exception was handled...");
}
System.out.println("This is the end of the program...");
}
}
现在输出已经改变:
异常已处理...这是程序的结尾...进程已完成,退出代码为 0 进程已完成,退出代码为 0
在这种情况下,程序正确完成,我们的消息显示在屏幕上。程序的正确终止由进程结束时的代码 0 表示,而不正确的是 — 1。使用 try...catch 块时,首先执行 try 和 catch 语句之间的所有语句。如果try块中发生异常,则正常执行顺序停止并继续执行catch语句。因此,当程序执行到numbers[7]=8时;行,程序将停止并转到 catch 块。在我们的例子中,我们声明了 Exception 类型的变量 myEx。这是所有异常的基类,因此它们可以不同。例如,有些异常会导致堆栈溢出、超出数组索引(如我们的例子中)指向 Null 等。如果我们没有猜测异常的类型,那么程序也会错误地终止。但是,为了示例简单起见,我们选择了 Exception 类型,它是所有异常的基类。所以catch语句(Exception myEx)将处理几乎所有异常。处理这种情况下的异常 在 catch 块完成后,程序继续其工作,执行 catch 块之后的所有其他指令。如果您想查看发生的异常,可以让程序打印方法调用的堆栈跟踪。这就是 JVM 在发生未捕获的异常时所做的事情:它停止程序的执行,并在执行 finally 块的代码(如果存在)后打印堆栈跟踪。
public class TryTest {
public static void main(String[] args) {
try {
int[] myArray = new int[5];
myArray[7] = 8;
System.out.println(myArray[7]);
} catch (Exception myEx) {
myEx.printStackTrace();
}
System.out.println("This is the end of the program...");
}
}
在这种情况下,处理异常归结为使用Exception类中定义的printStackTrace()方法将错误跟踪堆栈打印到控制台。
java.lang.ArrayIndexOutOfBoundsException:索引 7 在 days.TryTest.main(TryTest.java:7) 超出长度 5 的范围,这是程序的结尾...进程已完成,退出代码为 0
但是,程序正确退出。
最后!抓到后
在示例和异常处理关键字的定义中,我们提到了finally 块。它是可选的,但如果存在,则无论 try 块的结果如何,都会执行它。让我们将异常类型更改为 NullPointerException。public class TryTest {
public static void main(String[] args) {
try {
int[] myArray = new int[5];
myArray[7] = 8;
System.out.println(myArray[7]);
} catch (NullPointerException myEx) {
System.out.println("The exception was handled...");
}
finally{
System.out.println(" finally");
}
System.out.println("This is the end of the program...");
}
}
这是输出:
线程“main”中出现异常 java.lang.ArrayIndexOutOfBoundsException:索引 7 在 days.TryTest.main(TryTest.java:7) 超出长度 5 的范围最终进程完成,退出代码 1
顺便说一下,我们可以指定正确的异常类型。这里是 IndexOutOfBoundsException。
public class TryTest {
public static void main(String[] args) {
try {
int[] myArray = new int[5];
myArray[7] = 8;
System.out.println(myArray[7]);
} catch (IndexOutOfBoundsException myEx) {
System.out.println("The exception was handled...");
}
finally{
System.out.println(" finally");
}
System.out.println("This is the end of the program...");
}
}
在这种情况下,输出将如下:
异常已处理...最后这是程序的结束...进程已完成,退出代码为 0
异常的工作原理是什么?
关键是所有这些词——catch、 throw、 throws 只能与 java.lang.Throwable 或其后代一起使用。例如,您可以这样做:public class MyClass {
public static void main(String[] args) {
try {
} catch (Throwable thr) {
}
}
}
但是,你不能这样做:
public class MyClass {
public static void main(String[] args) {
try {
} catch (String thr) {
}
}
}
GO TO FULL VERSION