什麼是 Java 中的矩陣/二維數組?
“矩陣是排列成固定數量的行和列的數字集合。” 通常這些是實數。一般來說,矩陣可以包含複數,但為了簡單起見,我們在這裡只使用整數。讓我們看看矩陣是什麼樣子的。這是一個具有 4 行和 4 列的矩陣的示例。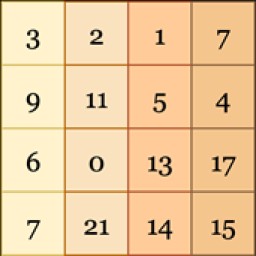
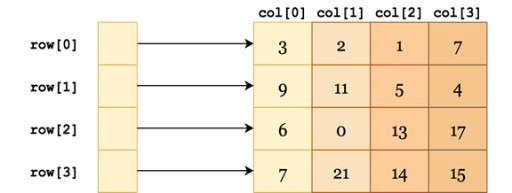
聲明並初始化一個二維數組
這裡有一些不同的方法,可以只聲明數組的大小,也可以在不提及大小的情況下對其進行初始化。
public class Matrices {
public static void main(String[] args) {
// declare & initialize 2D arrays for int and string
int[][] matrix1 = new int[2][2];
int matrix2[][] = new int[2][3];
//the size of matrix3 will be 4x4
int[][] matrix3 = { { 3, 2, 1, 7 },
{ 9, 11, 5, 4 },
{ 6, 0, 13, 17 },
{ 7, 21, 14, 15 } };
String[][] matrix4 = new String[2][2];
//the size of matrix5 will be 2x3
// 3 cols because at max there are 3 columns
String[][] matrix5 = { { "a", "lion", "meo" },
{ "jaguar", "hunt" } };
}
}
二維數組遍歷
我們都知道如何在 Java 中遍歷常規數組。對於二維數組也不難。我們通常為此使用嵌套的“for”循環。一些初學者可能會認為它是一些陌生的概念,但是只要您深入研究它,您就可以通過一些實踐來實現它。看看下面的片段。它只顯示每行對應的列數,以供您透徹理解。
public class MatrixTraversal {
public static void main(String[] args) {
int[][] matrix = new int[4][4];
for (int i = 0; i < matrix.length; i++)
{
// length returns number of rows
System.out.print("row " + i + " : ");
for (int j = 0; j < matrix[i].length; j++)
{
// here length returns # of columns corresponding to current row
System.out.print("col " + j + " ");
}
System.out.println();
}
}
}
輸出
第 0 行:col 0 col 1 col 2 col 3 第 1 行:col 0 col 1 col 2 col 3 第 2 行:col 0 col 1 col 2 col 3 第 3 行:col 0 col 1 col 2 col 3
如何在 Java 中打印二維數組?
熟悉二維數組遍歷後,讓我們看一下在 Java 中打印二維數組的幾種方法。使用嵌套的“for”循環
這是在 Java 中打印矩陣的最基本方法。
public class MatrixTraversal {
public static void printMatrix(int matrix[][])
{
for (int i = 0; i < matrix.length; i++)
{
// length returns number of rows
for (int j = 0; j < matrix[i].length; j++)
{
// here length returns number of columns corresponding to current row
// using tabs for equal spaces, looks better aligned
// matrix[i][j] will return each element placed at row ‘i',column 'j'
System.out.print( matrix[i][j] + "\t");
}
System.out.println();
}
}
public static void main(String[] args) {
int[][] matrix = { { 3, 2, 1, 7 },
{ 9, 11, 5, 4 },
{ 6, 0, 13, 17 },
{ 7, 21, 14, 15 } };
printMatrix(matrix);
}
}
輸出
3 2 1 7 9 11 5 4 6 0 13 17 7 21 14 15
使用“for-each”循環
這是使用“ foreach 循環”在 Java 中打印二維數組的另一種方法。這是 Java 提供的一種特殊類型的循環,其中 int[]row 將循環遍歷矩陣中的每一行。然而,變量“element”將包含通過行放置在列索引處的每個元素。
public class MatrixTraversal {
public static void printMatrix(int matrix[][]){
for (int [] row : matrix)
{
// traverses through number of rows
for (int element : row)
{
// 'element' has current element of row index
System.out.print( element + "\t");
}
System.out.println();
}
}
public static void main(String[] args) {
int[][] matrix = { { 3, 2, 1, 7 },
{ 9, 11, 5, 4 },
{ 6, 0, 13, 17 },
{ 7, 21, 14, 15 } };
printMatrix(matrix);
}
}
輸出
3 2 1 7 9 11 5 4 6 0 13 17 7 21 14 15
使用“Arays.toString()”方法
Java 中的Arrays.toString()方法,將傳遞給它的每個參數轉換為單個數組,並使用其內置方法打印它。我們創建了一個虛擬的 String 2D 數組來玩。同樣的方法也適用於整數數組。我們鼓勵您在鍛煉時練習它。
import java.util.Arrays;
public class MatrixTraversal {
public static void printMatrix(String matrix[][]){
for (String[] row : matrix) {
// convert each row to a String before printing
System.out.println(Arrays.toString(row));
}
}
public static void main(String[] args) {
String [][] matrix = { { "Hi, I am Karen" },
{ "I'm new to Java"},
{ "I love swimming" },
{ "sometimes I play keyboard"} };
printMatrix(matrix);
}
}
輸出
[嗨,我是凱倫] [我是 Java 的新手] [我喜歡游泳] [有時我會玩鍵盤]
GO TO FULL VERSION