Java中的集合是什么?
java中的集合被表示为一个容器,它将所有元素分组为一个单元。 例如,邮件文件夹(一组电子邮件)、电话簿(姓名到电话号码的映射)。什么是框架?
框架是一个基本基础或布局,您可以在其上使用提供的不同类和接口开始工作。 例如,Laravel 是最著名的 PHP 框架之一,它为您的应用程序提供了基本框架。Java 中的集合框架是什么?
所有对象都被分组为单个对象以及表示和提供操作集合的不同方法的体系结构。因此,Java 中的集合框架提供了已经实现的不同数据结构来存储数据和方法,并通过排序、搜索、删除和插入等功能来操作它们。 例如,您想要为某个随机公司实施一个系统,以基于先到先服务的原则改善其客户的服务。这也称为 FIFO(先进先出)实现。现在我们需要实现这个数据结构,然后用它来实现我们的目标。Collections框架为我们提供了一个Queue接口,我们只需要导入它,而不需要实现它,然后使用它,就完成了。 实现:您可以使用以下行导入所有集合:
import java.util.*;
如果要导入特定集合,请使用确切的包名称,例如:
import java.util.LinkedList;
Java 中集合框架的优点
它具有以下优点。- 已经实施(节省时间)。
- 性能效率(速度和质量)。
- 减少学习和使用新 API 的工作量。
集合框架的层次结构是什么?
现在让我们看看集合层次结构,但首先,我们需要了解该框架的基本组件。- 接口
- 类(实现)
- 算法
集合框架的层次结构
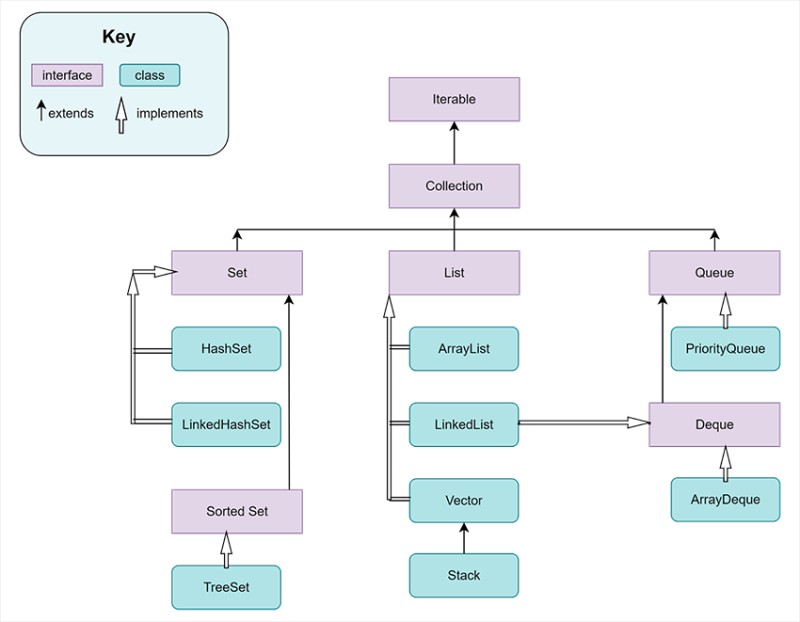
- Collection、Set、Queue 和 List 都是接口。Set、Queue 和 List由 Collection 接口扩展。
- PriorityQueue、HashSet、LinkedList 和 Stack 都是类或这些接口的实现。
- 一个类不强制只实现 一个接口。例如,LinkedList 还实现了 Deque 接口。
收藏类型
Java集合框架中有很多类型的集合来减少我们的工作量。以下是一些集合的列表:- 数组列表类
- 链表类
- 列表界面
- 设置界面
- 队列接口
- 地图界面
- 优先队列类
- HashMap类
- 类似的接口
- LinkedHashMap 类
- 树图类
- 哈希表
集合接口
这里我们将讨论一些常见的集合接口,然后讨论类实现的一些方法。采集接口
这是集合框架的基础,因为它提供了所有必要的实现方法。Map 是唯一没有实现它的数据结构,但其余的都实现了它的方法。该接口具有用于了解集合的大小、集合中是否存在对象、从集合中添加或删除对象的方法。可迭代接口
它是 Collections 框架的根接口,因为它是由所有类实现的 Collection 接口扩展的。它返回特定集合的迭代器以对其进行迭代。队列接口
队列用于保存元素,但不能对其进行处理。实现了基本的集合操作,它还提供了额外的插入和提取方法。设置界面
集合用于保存其中唯一的元素。它从不包含重复的元素,并对数学集合抽象进行建模来表示集合,例如机器上运行的进程。列表界面
列表是一个有序集合,有时称为序列,它可以在其中保存重复元素。它为用户提供更新或删除特定元素的控制,通过使用其整数索引值在特定点插入元素。LinkedList和ArrayList是List接口的实现类。双端队列接口
Deque代表双端队列,这意味着我们可以在两端执行操作。我们可以从两端插入和删除元素。Deque 接口扩展了队列接口。ArrayDeque 和 LinkedList 都实现了 Deque 接口。它提供了插入、删除和从两端检查实例的方法。地图界面
Map 接口也是 Collections 框架的一部分,但它不扩展 Collection 接口。它用于存储键值对。它的主要实现是HashMap、TreeMap和LinkesHashMap,它们在某些方面与HashSet、TreeSet和LinkedHashSet类似。它始终包含唯一的键,但值可以重复。当您需要根据键添加、删除或搜索项目时,它非常有用。它为我们提供了put、get、remove、size、empty等基本方法。这些接口的常用方法
现在我们来看看这个框架中除Map接口之外的不同类的实现所提供的一些常用方法。方法 | 描述 |
---|---|
公共布尔加法(E e) | 用于向集合中插入一个元素 |
公共布尔删除(对象元素) | 用于从集合中删除一个元素 |
公共 int 大小() | 返回集合中元素的数量 |
公共布尔包含(对象元素) | 用于搜索元素 |
公共布尔 isEmpty() | 检查集合是否为空 |
公共布尔等于(对象元素) | 检查平等性 |
集合类
众所周知,框架有不同的接口,由其中的许多类实现。现在我们来看看一些常用的类。链表
它是最常用的数据结构,它实现了双向链表来存储其中的元素。它可以存储重复的元素。它实现了由Queue接口和List接口扩展的Dequeue接口。它不同步。现在让我们看看如何使用 LinkedList 解决上面讨论的问题(先进先出概念)。问题是以顾客到达的方式(即先进先出)为顾客提供服务。例子
import java.util.*;
public class LinkedListExample {
public static void main(String[] args) {
Queue<String> customerQueue = new LinkedList<String>();
//Adding customers to the Queue as they arrived
customerQueue.add("John");
customerQueue.add("Angelina");
customerQueue.add("Brooke");
customerQueue.add("Maxwell");
System.out.println("Customers in Queue:"+customerQueue);
//element() => returns head of the queue
//we will see our first customer and serve him
System.out.println("Head of the queue i.e first customer: "+customerQueue.element());
//remove () method =>removes first element(customer) from the queue i.e the customer is served so remove him to see next
System.out.println("Element removed from the queue: "+customerQueue.remove());
//poll () => removes and returns the head
System.out.println("Poll():Returned Head of the queue: "+customerQueue.poll());
//print the remaining customers in the Queue
System.out.println("Final Queue:"+customerQueue);
}
}
输出
队列中的客户:[John、Angelina、Brooke、Maxwell] 队列头,即第一个客户:John 从队列中删除的元素:John Poll():Returned 队列头:Angelina 最终队列:[Brooke、Maxwell]
数组列表
它只是实现了 List 接口。它维护插入顺序并使用动态数组来存储不同数据类型的元素。元素可以重复。它也是非同步的并且可以存储空值。现在让我们看看它的不同方法...当我们不知道需要插入多少记录或元素时,这些方法很有用。让我们举一个图书馆的例子,我们不知道我们需要保存多少本书。所以每当我们有一本书时,我们需要将它插入到ArrayList中。例子
public class ArrayListExample {
public static void main(String args[]) {
// Creating the ArrayList
ArrayList<String> books = new ArrayList<String>();
// Adding a book to the list
books.add("Absalom, Absalom!");
// Adding a book in array list
books.add("A Time to Kill");
// Adding a book to the list
books.add("The House of Mirth");
// Adding a book to the list
books.add("East of Eden");
// Traversing the list through Iterator
Iterator<String> itr = books.iterator();
while (itr.hasNext()) {
System.out.println(itr.next());
}
}
}
输出
押沙龙,押沙龙!杀死伊甸园东欢乐屋的时刻
哈希集
它实现 Set 接口并且从不包含重复值。它实现了用于存储值的哈希表。它还允许空值。它从不维护插入顺序,但为add、remove、size和contains方法提供恒定的时间性能。最适合搜索操作,并且不同步。例子
import java.util.*;
class HashSetExample{
public static void main(String args[]){
//creating HashSet and adding elements to it
HashSet<Integer> hashSet=new HashSet();
hashSet.add(1);
hashSet.add(5);
hashSet.add(4);
hashSet.add(3);
hashSet.add(2);
//getting an iterator for the collection
Iterator<Integer> i=hashSet.iterator();
//iterating over the value
while(i.hasNext()) {
System.out.println(i.next());
}
}
}
输出
1 2 3 4 5
如您所见,它不维护插入顺序。
数组双端队列
它实现了 Deque 接口,因此允许从两端进行操作。它不允许空值。当实现为 Stack 和 LinkedList 时,它比 Stack 和 LinkedList 更快。ArrayDeque 没有大小限制,因为它可以根据要求增长和缩小。它是不同步的,这意味着它不是线程安全的。为了保持线程安全,我们必须实现一些外部逻辑。例子
import java.util.*;
public class ArrayDequeExample {
public static void main(String[] args) {
//creating Deque and adding elements
Deque<String> deque = new ArrayDeque<String>();
//adding an element
deque.add("One");
//adding an element at the start
deque.addFirst("Two");
//adding an element at the end
deque.addLast("Three");
//traversing elements of the collection
for (String str : deque) {
System.out.println(str);
}
}
}
输出
二一三
哈希映射
它是哈希表支持的 Map 接口的实现。它存储键值对。它不允许空值。它不同步。它从不保证插入顺序。它为get和put等方法提供恒定的时间性能。其性能取决于两个因素——初始容量和负载系数。容量是哈希表中存储桶的数量,因此初始容量是创建时分配的存储桶的数量。负载因子是衡量哈希表在容量增加之前可以填充多少内容的指标。rehash方法用于增加容量,主要是桶数加倍。例子
import java.util.*;
public class HashMapExample{
public static void main(String args[]){
//creating a HashMap
HashMap<Integer,String> map=new HashMap<Integer,String>();
//putting elements into the map
map.put(1,"England");
map.put(2,"USA");
map.put(3,"China");
//get element at index 2
System.out.println("Value at index 2 is: "+map.get(2));
System.out.println("iterating map");
//iterating the map
for(Map.Entry m : map.entrySet()){
System.out.println(m.getKey()+" "+m.getValue());
}
}
}
输出
索引 2 处的值为:中国迭代地图 1 英国 2 美国 3 中国
算法
Collections框架为我们提供了不同的算法来将不同的操作应用于集合。这里我们将看看这些算法涵盖了哪些主要操作。它包含与以下相关的算法:- 排序
- 搜寻中
- 洗牌
- 常规数据操作
- 作品
- 寻找极值
排序
排序算法根据排序关系对列表进行重新排序。提供了两种形式的关系。- 自然排序
- 比较订购
自然排序
在自然排序中,列表根据其元素进行排序。比较订购
在这种排序形式中,附加参数(比较器)与列表一起传递。使用稍微优化的合并排序算法进行排序,该算法快速且稳定,因为它保证了 n log(n) 运行时间,并且不会对相等的元素进行重新排序。我们将使用 ArrayList 中的相同示例来演示排序。例子
import java.util.*;
public class SortingExample{
public static void main(String args[]){
//Creating arraylist
ArrayList<String> books=new ArrayList<String>();
//Adding a book to the arraylist
books.add("A Time to Kill");
//Adding a book to the arraylist
books.add("Absalom, Absalom!");
//Adding a book to the arraylist
books.add("The House of Mirth");
//Adding a book to the arraylist
books.add("East of Eden");
//Traversing list through Iterator before sorting
Iterator itrBeforeSort=books.iterator();
while(itrBeforeSort.hasNext()){
System.out.println(itrBeforeSort.next());
}
//sorting the books
Collections.sort(books);
System.out.println("After sorting the books");
//Traversing list through Iterator after sorting
Iterator itr=books.iterator();
while(itr.hasNext()){
System.out.println(itr.next());
}
}
}
输出
杀押沙龙的时刻到了,押沙龙!伊甸园东边的欢乐之家 在整理书籍后,杀死押沙龙的时刻,押沙龙!伊甸园之东欢乐之家
GO TO FULL VERSION