안녕! 오늘 우리는 instanceof 연산자 에 대해 이야기하고 , 사용 방법의 예를 고려하고, 작동 방식의 일부 측면을 다룰 것입니다.) CodeGym의 초기 수준에서 이 연산자를 이미 접했습니다. 왜 필요한지 기억하십니까? 그렇지 않다면 걱정할 필요가 없습니다. 함께 기억합시다. instanceof 연산자 는 X 변수 가 참조하는 개체가 일부 Y 클래스를 기반으로 생성되었는지 여부를 확인하는 데 필요합니다 . 간단하게 들립니다. 이 주제로 돌아온 이유는 무엇입니까? 우선, 이제 Java의 상속 메커니즘과 OOP의 다른 원칙에 대해 잘 알고 있기 때문입니다. 이제 instanceof 연산자 가 훨씬 더 명확해지며 사용 방법에 대한 고급 예제를 살펴보겠습니다. 갑시다!
검사 결과가 참 이면 instanceof 연산자가 참을 반환 하고 표현식이 거짓이면 거짓을 반환한다는 사실을 기억할 것입니다 . 따라서 모든 종류의 조건식( if…else )에서 주로 발생합니다. 몇 가지 더 간단한 예부터 시작하겠습니다.
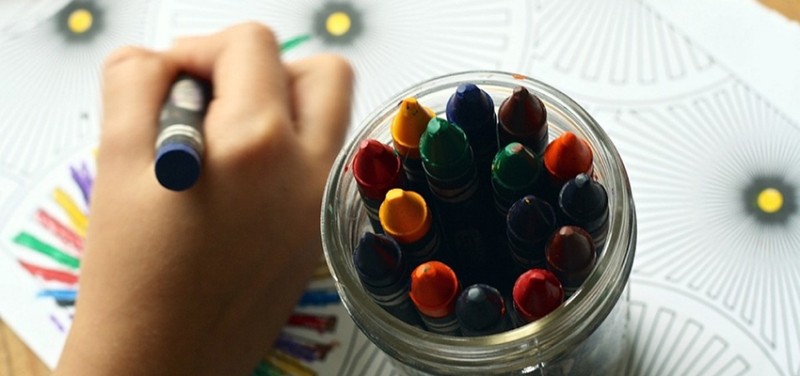
public class Main {
public static void main(String[] args) {
Integer x = new Integer(22);
System.out.println(x instanceof Integer);
}
}
콘솔에 무엇이 표시될 것이라고 생각하십니까? 음, 여기에서 분명합니다. :) x 개체는 Integer이므로 결과는 true 입니다 . 콘솔 출력: True 문자열 인지 확인해 봅시다 .
public class Main {
public static void main(String[] args) {
Integer x = new Integer(22);
System.out.println(x instanceof String); // Error!
}
}
오류가 발생했습니다. 그리고 주의하십시오: 컴파일러는 코드를 실행하기 전에 오류를 생성했습니다! Integer 와 String이 자동으로 서로 변환될 수 없으며 상속을 통해 관련이 없음을 즉시 확인했습니다 . 결과적으로 Integer 객체는 String 기반으로 생성되지 않습니다 . 이것은 편리하고 이상한 런타임 오류를 피하는 데 도움이 되므로 컴파일러가 여기에서 우리를 도왔습니다 :) 이제 더 어려운 예제를 고려해 보겠습니다. 상속에 대해 언급했으므로 다음과 같은 소규모 클래스 시스템으로 작업해 보겠습니다.
public class Animal {
}
public class Cat extends Animal {
}
public class MaineCoon extends Cat {
}
객체가 클래스의 인스턴스인지 여부를 확인할 때 instanceof가 어떻게 작동하는지 이미 알고 있지만 부모-자식 관계를 고려하면 어떻게 될까요? 예를 들어, 다음 표현식이 무엇을 산출할 것이라고 생각하십니까?
public class Main {
public static void main(String[] args) {
Cat cat = new Cat();
System.out.println(cat instanceof Animal);
System.out.println(cat instanceof MaineCoon);
}
}
출력: 참 거짓 대답해야 할 주요 질문은 instanceof가 '클래스를 기반으로 생성된 객체'를 정확히 어떻게 해석하는가입니다. ' cat instanceof Animal ' 은 true 로 평가되지만 확실히 그 문구에서 결함을 찾을 수 있습니다. Animal 클래스를 기반으로 Cat 개체가 생성되는 이유는 무엇입니까 ? 자체 클래스만으로 생성되지 않습니까? 대답은 충분히 간단하며 이미 생각했을 수도 있습니다. 객체를 생성할 때 생성자가 호출되고 변수가 초기화되는 순서를 기억하십시오. 우리는 이미 클래스 생성자에 대한 기사 에서 이 주제를 다루었습니다 . 다음은 해당 강의의 예입니다.
public class Animal {
String brain = "Initial value of brain in the Animal class";
String heart = "Initial value of heart in the Animal class";
public static int animalCount = 7700000;
public Animal(String brain, String heart) {
System.out.println("Animal base class constructor is running");
System.out.println("Have the variables of the Animal class already been initialized?");
System.out.println("Current value of static variable animalCount = " + animalCount);
System.out.println("Current value of brain in the Animal class = " + this.brain);
System.out.println("Current value of heart in the Animal class = " + this.heart);
System.out.println("Have the variables of the Cat class already been initialized?");
System.out.println("Current value of static variable catCount = " + Cat.catCount);
this.brain = brain;
this.heart = heart;
System.out.println("Animal base class constructor is done!");
System.out.println("Current value of brain = " + this.brain);
System.out.println("Current value of heart = " + this.heart);
}
}
public class Cat extends Animal {
String tail = "Initial value of tail in the Cat class";
static int catCount = 37;
public Cat(String brain, String heart, String tail) {
super(brain, heart);
System.out.println("The Cat class constructor has started (The Animal constructor already finished)");
System.out.println("Current value of static variable catCount = " + catCount);
System.out.println("Current value of tail = " + this.tail);
this.tail = tail;
System.out.println("Current value of tail = " + this.tail);
}
public static void main(String[] args) {
Cat cat = new Cat("Brain", "Heart", "Tail");
}
}
IDE에서 실행하면 콘솔 출력은 다음과 같이 표시됩니다. Animal 기본 클래스 생성자가 실행 중입니다. Animal 클래스의 변수가 이미 초기화되었습니까? 정적 변수 animalCount의 현재 값 = 7700000 Animal 클래스의 뇌의 현재 값 = Animal 클래스의 뇌의 초기 값 Animal 클래스의 심장의 현재 값 = Animal 클래스의 심장의 초기 값 이미 Cat 클래스의 변수를 가지고 있음 초기화 되었습니까? 정적 변수 catCount의 현재 값 = 37 Animal 기본 클래스 생성자가 완료되었습니다! brain의 현재값 = Brain 현재값 heart = Heart cat 클래스 생성자가 시작됨 (Animal 생성자는 이미 완료됨) 정적 변수 catCount의 현재값 = 37 tail의 현재값 = Cat 클래스의 tail의 초기값 tail의 현재값 = 꼬리 이제 기억나시나요?:) 기본 클래스의 생성자는 기본 클래스가 있는 경우 객체를 생성할 때 항상 먼저 호출됩니다. instanceof 연산자 는 A 개체가 B 클래스 를 기반으로 생성되었는지 여부를 확인하려고 할 때 이 원칙을 따릅니다 . 기본 클래스 생성자가 호출되면 의심의 여지가 없습니다. 두 번째 확인으로 모든 것이 더 간단해집니다.
System.out.println(cat instanceof MaineCoon);
MaineCoon 생성자는 Cat 개체가 생성될 때 호출되지 않았습니다 . 결국 MaineCoon은 Cat 의 후손 이지 조상이 아닙니다. 그리고 Cat 의 템플릿이 아닙니다 . 좋아, 나는 우리가 그것에 대해 분명하다고 생각한다. 하지만 이렇게 하면 어떻게 될까요?:
public class Main {
public static void main(String[] args) {
Cat cat = new MaineCoon();
System.out.println(cat instanceof Cat);
System.out.println(cat instanceof MaineCoon);
}
}
흠... 더 어렵네요. 그것에 대해 이야기합시다. MaineCoon 개체를 할당한 Cat 변수가 있습니다 . 그건 그렇고, 왜 작동합니까? 우리가 할 수 있어, 그렇지? 그래 우리는 할 수있어. 결국 모든 MaineCoon은 고양이입니다. 완전히 명확하지 않은 경우 확장 기본 유형의 예를 기억하십시오.
public class Main {
public static void main(String[] args) {
long x = 1024;
}
}
숫자 1024 는 짧습니다 . 수용하기에 충분한 바이트가 있기 때문에 긴 변수 에 쉽게 맞습니다 (인형의 예를 기억하십니까?). 자손 개체는 항상 조상 변수에 할당될 수 있습니다. 지금은 이것만 기억하고 다음 단원에서는 작동 방식을 분석할 것입니다. 그래서 우리의 예는 무엇을 출력합니까?
Cat cat = new MaineCoon();
System.out.println(cat instanceof Cat);
System.out.println(cat instanceof MaineCoon);
instanceof는 무엇을 확인합니까? Cat 변수 또는 MaineCoon 개체? 대답은 이 질문이 실제로 간단하다는 것입니다. 연산자의 정의를 다시 읽으면 됩니다. X 변수 가 참조하는 개체가 일부 Y 클래스 를 기반으로 생성되었는지 여부를 확인하려면 instanceof 연산자가 필요합니다 . instanceof 연산자 는 변수 유형이 아니라 객체의 출처를 테스트합니다. 따라서 이 예에서 프로그램은 두 경우 모두 true를 표시합니다. MaineCoon 개체가 있습니다 . 분명히 MaineCoon 클래스를 기반으로 만들었지 만 Cat을 기반으로 만들었습니다.학부모 수업도!
더 읽어보기: |
---|
GO TO FULL VERSION