"안녕하세요! 오늘 수업에서는 Java( Java I/O )의 입력 및 출력 스트림에 대한 대화를 계속할 것입니다. 이 주제에 대한 첫 번째 수업도 아니고 확실히 마지막도 아닐 것입니다
:) 자바 언어는 I/O로 작업할 수 있는 많은 방법을 제공합니다. 이 기능을 구현하는 클래스가 상당히 많기 때문에 처음부터 혼동하지 않도록 여러 레슨으로 나누었습니다. :) 수업에서 우리는
다음은 다음을 사용하여 파일에서 데이터를 읽는 모습입니다
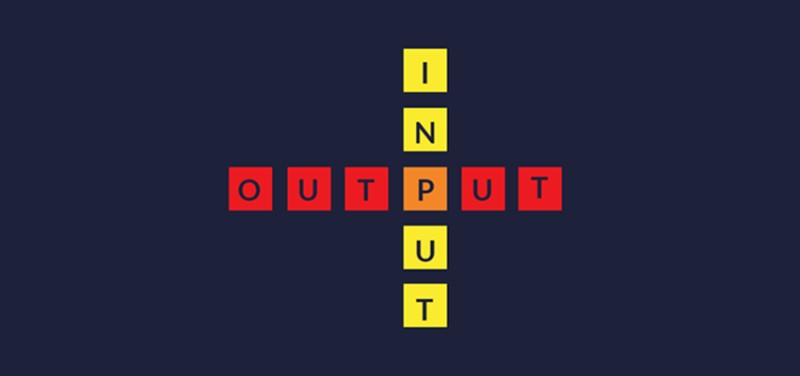
BufferedReader
, 및 추상 클래스와 여러 하위 클래스에 대해 다루었습니다 . 오늘 우리는 , , 의 세 가지 새로운 클래스 InputStream
를 고려할 것입니다 . OutputStream
FileInputStream
FileOutputStream
BufferedInputStream
FileOutputStream 클래스
클래스 의 주요 목적은FileOutputStream
파일에 바이트를 쓰는 것입니다. 복잡한 것은 없습니다 :)는 추상 클래스 FileOutputStream
의 구현 중 하나입니다 OutputStream
. 생성자에서 이 클래스의 개체는 대상 파일(바이트를 기록해야 하는 위치) 또는 개체에 대한 경로를 사용합니다 File
. 각각의 예를 살펴보겠습니다.
public class Main {
public static void main(String[] args) throws IOException {
File file = new File("C:\\Users\\Username\\Desktop\\test.txt");
FileOutputStream fileOutputStream = new FileOutputStream(file);
String greetings = "Hi! Welcome to CodeGym — The best site for would-be programmers!";
fileOutputStream.write(greetings.getBytes());
fileOutputStream.close();
}
}
개체를 만들 때 File
원하는 경로를 생성자에 전달했습니다. 미리 생성할 필요는 없습니다. 존재하지 않으면 프로그램이 생성합니다. 추가 객체를 생성하지 않고 경로와 함께 문자열을 전달하기만 하면 얻을 수 있습니다.
public class Main {
public static void main(String[] args) throws IOException {
FileOutputStream fileOutputStream = new FileOutputStream("C:\\Users\\Username\\Desktop\\test.txt");
String greetings = "Hi! Welcome to CodeGym — The best site for would-be programmers!";
fileOutputStream.write(greetings.getBytes());
fileOutputStream.close();
}
}
두 경우 모두 결과는 동일합니다. 파일을 열고 다음을 볼 수 있습니다.
Hi! Welcome to CodeGym — The best site for would-be programmers!
그러나 여기에는 한 가지 뉘앙스가 있습니다. 위 예제의 코드를 연속으로 여러 번 실행해 보십시오. 그런 다음 파일을 보고 다음 질문에 답하십시오. 파일에 몇 줄이 있습니까? 딱 하나만. 하지만 코드를 여러 번 실행했습니다. 매번 데이터를 덮어쓰는 것으로 나타났습니다. 이전 데이터는 새 데이터로 대체됩니다. 그것이 우리에게 적합하지 않고 파일에 순차적으로 작성해야 하는 경우 어떻게 해야 합니까? 인사말을 파일에 연속으로 세 번 쓰고 싶다면 어떻게 해야 할까요? 모두 매우 간단합니다. 언어는 각 경우에 어떤 행동이 필요한지 알 수 없기 때문에 FileOutputStream
구성자는 추가 매개변수를 취할 수 있습니다.boolean append
. 값이 true이면 데이터가 파일 끝에 기록됩니다. false이면(기본값은 false임) 이전 데이터가 모두 지워지고 새 데이터로 대체됩니다. 수정된 코드를 세 번 실행하여 이를 확인합니다.
public class Main {
public static void main(String[] args) throws IOException {
FileOutputStream fileOutputStream = new FileOutputStream("C:\\Users\\Username\\Desktop\\test.txt", true);
String greetings = "Hi! Welcome to CodeGym — The best site for would-be programmers!\r\n";
fileOutputStream.write(greetings.getBytes());
fileOutputStream.close();
}
}
파일 내용:
Hi! Welcome to CodeGym — The best site for would-be programmers!
Hi! Welcome to CodeGym — The best site for would-be programmers!
Hi! Welcome to CodeGym — The best site for would-be programmers!
이제 다릅니다! I/O 클래스를 사용할 때 이 기능을 잊지 마십시오. 내 데이터가 파일에서 어떻게 사라지는지 이해하려고 몇 시간 동안 머리를 쥐어짜고 작업에 몇 시간을 보냈던 때가 있었습니다 :) 물론 다른 I/O 수업과 마찬가지로 이 방법을 사용하는 것을 잊지 close()
마세요 리소스를 확보합니다.
FileInputStream 클래스
에는FileInputStream
파일에서 바이트를 읽는 반대 목적이 있습니다. FileOutputStream
상속 과 마찬가지로 OutputStream
이 클래스는 InputStream
추상 클래스에서 파생됩니다. " test.txt " 파일 에 몇 줄의 텍스트를 작성합니다 .
"So close no matter how far
Couldn't be much more from the heart
Forever trusting who we are
And nothing else matters"
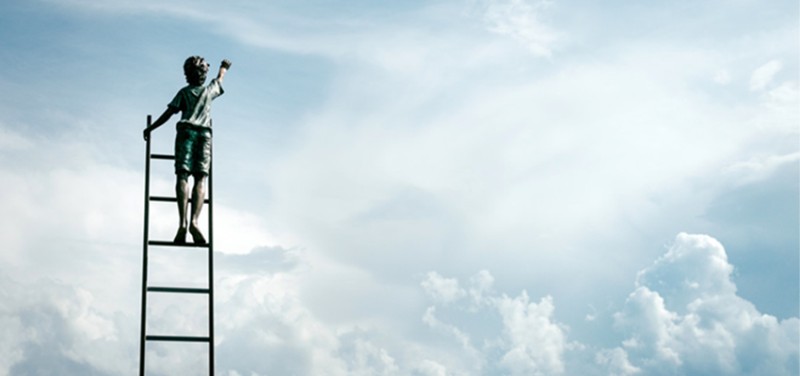
FileInputStream
.
public class Main {
public static void main(String[] args) throws IOException {
FileInputStream fileInputStream = new FileInputStream("C:\\Users\\Username\\Desktop\\test.txt");
int i;
while((i=fileInputStream.read())!= -1){
System.out.print((char)i);
}
}
}
파일에서 한 바이트를 읽고 읽은 바이트를 문자로 변환하여 콘솔에 표시합니다. 다음은 콘솔 출력입니다.
So close no matter how far
Couldn't be much more from the heart
Forever trusting who we are
And nothing else matters
BufferedInputStream 클래스
지난 수업에서 얻은 지식을 바탕으로 클래스가 필요한 이유BufferedInputStream
와 비교했을 때 어떤 이점이 있는지 쉽게 말할 수 있을 것 같습니다 FileInputStream
:) 버퍼링된 스트림을 이미 접했으므로 계속 읽기 전에 추측(또는 기억)해 보십시오 :) 버퍼링된 스트림은 주로 I/O를 최적화하는 데 필요합니다. 파일에서 읽기와 같이 데이터 소스에 액세스하는 것은 성능 측면에서 비용이 많이 드는 작업이며 각 바이트를 읽기 위해 파일에 액세스하는 것은 낭비입니다. 그렇기 때문에 BufferedInputStream
한 번에 한 바이트가 아니라 블록 단위로 데이터를 읽고 임시로 특수 버퍼에 저장합니다. 이렇게 하면 파일에 액세스하는 횟수를 줄여 프로그램을 최적화할 수 있습니다. 이것이 어떻게 보이는지 봅시다:
public class Main {
public static void main(String[] args) throws IOException {
FileInputStream fileInputStream = new FileInputStream("C:\\Users\\Username\\Desktop\\test.txt");
BufferedInputStream bufferedInputStream = new BufferedInputStream(fileInputStream, 200);
int i;
while((i = bufferedInputStream.read())!= -1){
System.out.print((char)i);
}
}
}
여기서 우리는 BufferedInputStream
객체를 만들었습니다. 그 생성자는 클래스의 인스턴스 InputStream
나 그 자손 중 하나를 취하므로 그렇게 FileInputStream
할 것입니다. 추가 인수로 버퍼 크기(바이트)를 사용합니다. 이 인수 덕분에 파일에서 한 번에 한 바이트가 아니라 한 번에 200바이트씩 데이터를 읽을 수 있습니다! 파일 액세스 횟수를 얼마나 줄였는지 상상해 보십시오. 성능을 비교하려면 큰 텍스트 파일(몇 메가바이트의 텍스트)을 가져와 FileInputStream
및 를 사용하여 읽고 콘솔에 출력하는 데 걸리는 시간(밀리초)을 비교할 수 있습니다 BufferedInputStream
. 다음은 두 옵션을 모두 보여주는 코드입니다.
public class Main {
public static void main(String[] args) throws IOException {
Date date = new Date();
FileInputStream fileInputStream = new FileInputStream("C:\\Users\\Username\\Desktop\\textBook.rtf");
BufferedInputStream bufferedInputStream = new BufferedInputStream(fileInputStream);
int i;
while((i = bufferedInputStream.read())!= -1){
System.out.print((char)i);
}
Date date1 = new Date();
System.out.println((date1.getTime() - date.getTime()));
}
}
public class Main {
public static void main(String[] args) throws IOException {
Date date = new Date();
FileInputStream fileInputStream = new FileInputStream("C:\\Users\\Username\\Desktop\\26951280.rtf");
int i;
while((i = fileInputStream.read())!= -1){
System.out.print((char)i);
}
Date date1 = new Date();
System.out.println((date1.getTime() - date.getTime()));
}
}
내 컴퓨터에서 1.5MB 파일을 읽을 때 FileInputStream
~3500밀리초 안에 작업을 완료했지만 BufferedInputStream
~1700밀리초 만에 처리했습니다. 보시다시피 버퍼링된 스트림은 작업을 최적화하여 절반으로 줄였습니다! :) I/O 수업은 계속 공부하겠습니다. 곧 뵙겠습니다!