์๋
! ์ค๋ ์์
์ ํธ์๋ฅผ ์ํด ๋ ๋ถ๋ถ์ผ๋ก ๋๋ฉ๋๋ค. ์ฐ๋ฆฌ๋ ์ด์ ์ ๋ค๋ฃจ์๋ ๋ช ๊ฐ์ง ์ค๋๋ ์ฃผ์ ๋ฅผ ๋ฐ๋ณตํ๊ณ ๋ช ๊ฐ์ง ์๋ก์ด ๊ธฐ๋ฅ์ ๊ณ ๋ คํ ๊ฒ์
๋๋ค :) ์ฒซ ๋ฒ์งธ ํญ๋ชฉ๋ถํฐ ์์ํ๊ฒ ์ต๋๋ค. ๋น์ ์ ์ด๋ฏธ ์ฌ๋ฌ ๋ฒ ๊ฐ์ ์์
์ํ์ต๋๋ค
๋ฌผ๋ก
์ด ์ผ์ด ์ผ์ด๋๋ ค๋ฉด ๋ฌด์์ด ํ์ํฉ๋๊น? ์ฐ์
BufferedReader
. ๋๋ ๋น์ ์ด ์ด ๋ง์ ์์ ์๊ฐ์ด ์์๊ธฐ๋ฅผ ๋ฐ๋๋๋ค.
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
System.in
์์ธํ ์ฝ๊ธฐ ์ ์ ๊ฐ ๊ตฌ์ฑ์์( , InputStreamReader
, BufferedReader
)๊ฐ ๋ด๋นํ๋ ๋ด์ฉ๊ณผ ํ์ํ ์ด์ ๋ฅผ ๊ธฐ์ตํด ๋ณด์ญ์์ค . ๊ธฐ์ตํ๋? ๊ทธ๋ ์ง ์๋ค๋ฉด ๊ฑฑ์ ํ ํ์๊ฐ ์์ต๋๋ค. :) ์์ด๋ฒ๋ฆฐ ๊ฒ์ด ์์ผ๋ฉด ๋
์ ํด๋์ค ์ ์ฉ ๊ฐ์์ธ ์ด ๊ฐ์๋ฅผ ๋ค์ ์ฝ์ด๋ณด์ธ์. ์ฐ๋ฆฌ๋ ๊ทธ๋ค ๊ฐ๊ฐ์ด ๋ฌด์์ ํ ์ ์๋์ง ๊ฐ๋ตํ๊ฒ ๊ธฐ์ตํ ๊ฒ์
๋๋ค. System.in
โ ์ด๊ฒ์ ํค๋ณด๋์์ ๋ฐ์ดํฐ๋ฅผ ์์ ํ๊ธฐ ์ํ ์คํธ๋ฆผ์
๋๋ค. โฏ์์น์ ์ผ๋ก ํ
์คํธ๋ฅผ ์ฝ๋ ๋ฐ ํ์ํ ๋
ผ๋ฆฌ๋ฅผ ๊ตฌํํ๋ ๋ฐ๋ ๊ทธ๊ฒ๋ง์ผ๋ก๋ ์ถฉ๋ถํฉ๋๋ค. ๊ทธ๋ฌ๋ ๊ธฐ์ตํ์๊ฒ ์ง๋ง System.in
๋ฐ์ดํธ๋ง ์ฝ์ ์ ์๊ณ ๋ฌธ์๋ ์ฝ์ ์ ์์ต๋๋ค.
public class Main {
public static void main(String[] args) throws IOException {
while (true) {
int x = System.in.read();
System.out.println(x);
}
}
}
์ด ์ฝ๋๋ฅผ ์คํํ๊ณ ํค๋ฆด ๋ฌธ์ "ะ"๋ฅผ ์
๋ ฅํ๋ฉด ์ถ๋ ฅ์ ๋ค์๊ณผ ๊ฐ์ต๋๋ค.
ะ
208
153
10
ํค๋ฆด ๋ฌธ์๋ ๋ฉ๋ชจ๋ฆฌ์์ 2๋ฐ์ดํธ๋ฅผ ์ฐจ์งํ๋ฉฐ ํ๋ฉด์ ํ์๋ฉ๋๋ค. ์ซ์ 10์ ์ค ๋ฐ๊ฟ ๋ฌธ์์ ์ญ์ง์ ํํ์
๋๋ค. ์๋ฅผ ๋ค์ด Enter ํค๋ฅผ ๋๋ฅด๋ฉด ๋ฉ๋๋ค. ๋ฐ์ดํธ ์ฝ๊ธฐ๋ ์ ๋ง ์ฆ๊ฒ๊ธฐ ๋๋ฌธ์ ์ฌ์ฉ์ด System.in
๊ทธ๋ฆฌ ํธ๋ฆฌํ์ง ์์ต๋๋ค. ํค๋ฆด ๋ฌธ์(๋ฐ ๊ธฐํ)๋ฅผ ์ฌ๋ฐ๋ฅด๊ฒ ์ฝ๊ธฐ ์ํด ๋ค์์ InputStreamReader
๋ํผ๋ก ์ฌ์ฉํฉ๋๋ค.
public class Main {
public static void main(String[] args) throws IOException {
InputStreamReader reader = new InputStreamReader(System.in);
while (true) {
int x = reader.read();
System.out.println(x);
}
}
}
๊ฐ์ ๋ฌธ์ "ะ"๋ฅผ ์
๋ ฅํ์ง๋ง ์ด๋ฒ์๋ ๊ฒฐ๊ณผ๊ฐ ๋ค๋ฆ
๋๋ค.
ะ
1049
10
InputStreamReader
2๋ฐ์ดํธ(208 ๋ฐ 153)๋ฅผ ๋จ์ผ ์ซ์ 1049๋ก ๋ณํํ์ต๋๋ค. ์ด๊ฒ์ด ๋ฌธ์๋ฅผ ์ฝ๋๋ค๋ ์๋ฏธ์
๋๋ค. 1049๋ ํค๋ฆด ๋ฌธ์ "ะ"์ ํด๋นํฉ๋๋ค. ์ฐ๋ฆฌ๋ ์ด๊ฒ์ด ์ฌ์ค์์ ์ฝ๊ฒ ํ์ ํ ์ ์์ต๋๋ค.
public class Main {
public static void main(String[] args) throws IOException {
char x = 1049;
System.out.println(x);
}
}
์ฝ์ ์ถ๋ ฅ:
ะ
๊ทธ๋ฆฌ๊ณ forBufferedReader
์ผ๋ฐ์ ์ผ๋ก BufferedAnythingYouWant
๋ฒํผ ํด๋์ค๋ ์ฑ๋ฅ์ ์ต์ ํํ๋ ๋ฐ ์ฌ์ฉ๋ฉ๋๋ค. ๋ฐ์ดํฐ ์์ค(ํ์ผ, ์ฝ์, ์น ๋ฆฌ์์ค)์ ์ก์ธ์คํ๋ ๊ฒ์ ์ฑ๋ฅ ๋ฉด์์ ์๋นํ ๋น์๋๋ค. ๋ฐ๋ผ์ ์ก์ธ์ค ์๋ฅผ ์ค์ด๊ธฐ ์ํด BufferedReader
ํน์ ๋ฒํผ์์ ๋ฐ์ดํฐ๋ฅผ ์ฝ๊ณ ์ถ์ ํ๊ณ ๊ฑฐ๊ธฐ์์ ๊ฐ์ ธ์ต๋๋ค. ๊ฒฐ๊ณผ์ ์ผ๋ก ๋ฐ์ดํฐ ์์ค์ ์ก์ธ์คํ๋ ํ์๊ฐ ๋ช ๋ฐฐ๋ ์ค์ด๋ญ๋๋ค! ์ ๋ ๋ค๋ฅธ BufferedReader
๊ธฐ๋ฅ๊ณผ ์ผ๋ฐ ์ ๋นํด ์ฅ์ ์ ๊ฐ๋ณ ์ซ์๊ฐ ์๋ ์ ์ฒด ๋ฐ์ดํฐ ํ์ ์ฝ๋ InputStreamReader
๋งค์ฐ ์ ์ฉํ ๋ฐฉ๋ฒ์
๋๋ค . readLine()
๋ฌผ๋ก ์ด๊ฒ์ ํฐ ํ
์คํธ๋ฅผ ๋ค๋ฃฐ ๋ ๋งค์ฐ ํธ๋ฆฌํฉ๋๋ค. ์ฝ๊ธฐ ๋ผ์ธ์ ๋ค์๊ณผ ๊ฐ์ต๋๋ค.
public class Main {
public static void main(String[] args) throws IOException {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
String s = reader.readLine();
System.out.println ("The user entered the following text:");
System.out.println(s);
reader.close();
}
}
BufferedReader+InputStreamReader is faster than InputStreamReader alone
The user entered the following text:
BufferedReader+InputStreamReader is faster than InputStreamReader alone
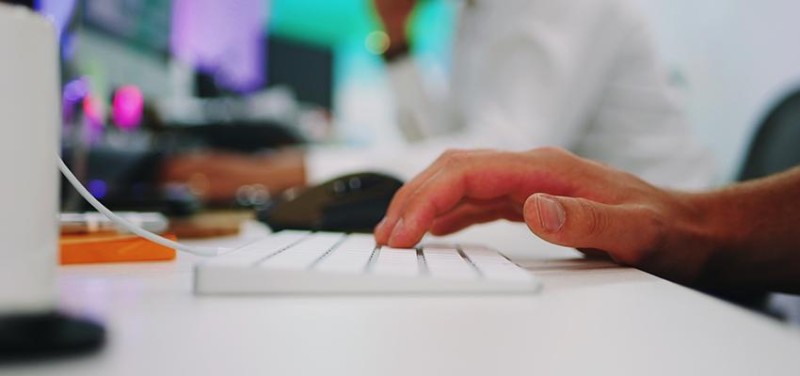
BufferedReader
๋งค์ฐ ์ ์ฐํฉ๋๋ค. ํค๋ณด๋ ์์
์๋ง ๊ตญํ๋์ง ์์ต๋๋ค. ์๋ฅผ ๋ค์ด ํ์ํ URL์ ํ๋
๊ธฐ์ ์ ๋ฌํ๊ธฐ๋ง ํ๋ฉด ์น์์ ์ง์ ๋ฐ์ดํฐ๋ฅผ ์ฝ์ ์ ์์ต๋๋ค.
public class URLReader {
public static void main(String[] args) throws Exception {
URL oracle = new URL("https://www.oracle.com/index.html");
BufferedReader in = new BufferedReader(
new InputStreamReader(oracle.openStream()));
String inputLine;
while ((inputLine = in.readLine()) != null)
System.out.println(inputLine);
in.close();
}
}
ํ์ผ ๊ฒฝ๋ก๋ฅผ ์ ๋ฌํ์ฌ ํ์ผ์์ ๋ฐ์ดํฐ๋ฅผ ์ฝ์ ์ ์์ต๋๋ค.
public class Main {
public static void main(String[] args) throws Exception {
FileInputStream fileInputStream = new FileInputStream("testFile.txt");
BufferedReader reader = new BufferedReader(new InputStreamReader(fileInputStream));
String str;
while ((str = reader.readLine()) != null) {
System.out.println (str);
}
reader.close();
}
}
System.out ๊ต์ฒด
์ด์ ์ด์ ์ ๋ค๋ฃจ์ง ์์ ํฅ๋ฏธ๋ก์ด ๊ธฐ๋ฅ์ ์ดํด๋ณด๊ฒ ์ต๋๋ค. ํ์คํ ๊ธฐ์ตํ๊ณ ์๋ฏ์ดSystem
ํด๋์ค์๋ ๋ ๊ฐ์ ์ ์ ํ๋ System.in
์ ๊ฐโฏ ์์ต๋๋ค System.out
. ์ด ์๋ฅ์ด ํ์ ๋ ์คํธ๋ฆผ ๊ฐ์ฒด์
๋๋ค.โฏSystem.in
๋ InputStream
. ๊ทธ๋ฆฌ๊ณ System.out
โฏ. PrintStream
_ ๋ฐ๋ก ์ง๊ธ ์ ๋ํด ์ด์ผ๊ธฐํ๊ฒ ์ต๋๋คโฏ System.out
. ํด๋์ค์ ์์ค ์ฝ๋ ๋ก ์ด๋ํ๋ฉด System
๋ค์๊ณผ ๊ฐ์ด ํ์๋ฉ๋๋ค.
public final class System {
โฆโฆโฆโฆโฆ...
public final static PrintStream out = null;
โฆโฆโฆโฆ
}
๋ฐ๋ผ์โฏ System.out
โฏ๋จ์ํ ํด๋์ค์ ์ผ๋ฐ ์ ์ ๋ณ์์
๋๋คSystem
. ๊ทธ๊ฒ์ ๋ํด ๋ง๋ฒ์ด ์์ต๋๋ค :) out
๋ณ์๋ ์ฐธ์กฐ์
๋๋ค PrintStream
. ๋ค์์ ํฅ๋ฏธ๋ก์ด ์ง๋ฌธ์
๋๋ค. ๊ฐ System.out.println()
์คํ๋ ๋ ์ถ๋ ฅ์ด ์ ํํ ๋ค๋ฅธ ๊ณณ์ด ์๋ ์ฝ์๋ก ์ด๋ํ๋ ์ด์ ๋ ๋ฌด์์
๋๊น? ๊ทธ๋ฆฌ๊ณ ์ด๊ฒ์ ์ด๋ป๊ฒ๋ ๋ฐ๊ฟ ์ ์์ต๋๊น? ์๋ฅผ ๋ค์ด ์ฝ์์์ ๋ฐ์ดํฐ๋ฅผ ์ฝ๊ณ ํ
์คํธ ํ์ผ์ ์ฐ๊ณ ์ถ๋ค๊ณ ๊ฐ์ ํฉ๋๋ค. System.out
์ถ๊ฐ ํ๋
๊ธฐ ๋ฐ ์์ฑ๊ธฐ ํด๋์ค๊ฐ ์๋ ์ฌ์ฉํ์ฌ ์ด๋ป๊ฒ๋ ๊ฐ๋จํ๊ฒ ๊ตฌํํ ์ ์์ต๋๊นโฏ ? ์ค์ ๋ก ๊ทธ๋ ์ต๋๋ค :) ๋ณ์ System.out
์ ์์์ด๊ฐ ํ์๋์ด ์์ด๋ ํ ์ ์์ต๋๋ค final
!โฏ 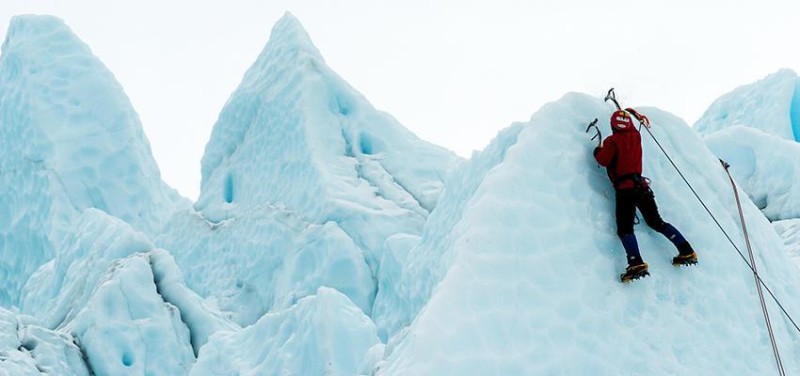
PrintStream
ํ์ฌ ๊ฐ์ฒด๋ฅผ ๋์ฒดํ ์ ๊ฐ์ฒด๊ฐ ํ์ํฉ๋๋ค. ์ ์ค์ ๋ ํ์ฌ ๊ฐ์ฒดSystem
ํด๋์ค๋ ๊ธฐ๋ณธ์ ์ผ๋ก ์ฐ๋ฆฌ์ ๋ชฉ์ ์ ๋ถํฉํ์ง ์์ต๋๋ค. ์ฝ์์ ๊ฐ๋ฆฌํต๋๋ค. ๋ฐ์ดํฐ์ "๋์"์ธ ํ
์คํธ ํ์ผ์ ๊ฐ๋ฆฌํค๋ ์ ํ์ผ์ ๋ง๋ค์ด์ผ ํฉ๋๋ค. ๋์งธ, ๋ณ์์ ์ ๊ฐ์ ํ ๋นํ๋ ๋ฐฉ๋ฒ์ ์ดํดํด์ผ ํฉ๋๋ค System.out
. ๋ณ์๊ฐ ๋ก ํ์๋์ด ์์ผ๋ฏ๋ก ๊ฐ๋จํ ๋์
์ฐ์ฐ์๋ฅผ ์ฌ์ฉํ ์ ์์ต๋๋ค final
. ๋์์ ๊ฑฐ๊พธ๋ก ์์
ํฉ์๋ค. ๊ณต๊ต๋กญ๊ฒ๋ System
ํด๋์ค์๋ ํ์ํ ๋ฉ์๋๊ฐ ์์ต๋๋ค setOut()
. ๊ฐ์ฒด๋ฅผ ๊ฐ์ ธ์ PrintStream
์ถ๋ ฅ ๋์์ผ๋ก ์ค์ ํฉ๋๋ค. ๊ทธ๊ฒ์ด ๋ฐ๋ก ์ฐ๋ฆฌ์๊ฒ ํ์ํ ๊ฒ์
๋๋ค! ๋จ์ ๊ฒ์ PrintStream
๊ฐ์ฒด๋ฅผ ๋ง๋๋ ๊ฒ์
๋๋ค. ์ด๊ฒ๋ ์ฝ์ต๋๋ค.
PrintStream filePrintStream = new PrintStream(new File("C:\\Users\\Username\\Desktop\\test.txt"));
์ ์ฒด ์ฝ๋๋ ๋ค์๊ณผ ๊ฐ์ต๋๋ค.
public class SystemRedirectService {
public static void main(String arr[]) throws FileNotFoundException
{
PrintStream filePrintStream = new PrintStream(new File("C:\\Users\\Username\\Desktop\\test.txt"));
/* Save the current value of System.out in a separate variable so that later
we can switch back to console output */
PrintStream console = System.out;
// Assign a new value to System.out
System.setOut(filePrintStream);
System.out.println("This line will be written to the text file");
// Restore the old value of System.out
System.setOut(console);
System.out.println("But this line will be output to the console!");
}
}
๊ฒฐ๊ณผ์ ์ผ๋ก ์ฒซ ๋ฒ์งธ ๋ฌธ์์ด์ ํ
์คํธ ํ์ผ์ ์์ฑ๋๊ณ ๋ ๋ฒ์งธ ๋ฌธ์์ด์ ์ฝ์์ ํ์๋ฉ๋๋ค :) ์ด ์ฝ๋๋ฅผ IDE์ ๋ณต์ฌํ์ฌ ์คํํ ์ ์์ต๋๋ค. ํ
์คํธ ํ์ผ์ ์ด๋ฉด ๋ฌธ์์ด์ด ์ฑ๊ณต์ ์ผ๋ก ์์ฑ๋ ๊ฒ์ ๋ณผ ์ ์์ต๋๋ค. :) ์ด๊ฒ์ผ๋ก ์์
์ด ๋๋ฌ์ต๋๋ค. ์ค๋ ์ฐ๋ฆฌ๋ ์คํธ๋ฆผ ๋ฐ ๋
์์ ํจ๊ป ์์
ํ๋ ๋ฐฉ๋ฒ์ ํ์ํ์ต๋๋ค. ์ฐ๋ฆฌ๋ ๊ทธ๋ค์ด ์๋ก ์ด๋ป๊ฒ ๋ค๋ฅธ์ง ๊ธฐ์ตํ๊ณ System.out
๊ฑฐ์ ๋ชจ๋ ์์
์์ ์ฌ์ฉํ ์ ๋ช ๊ฐ์ง ์๋ก์ด ๊ธฐ๋ฅ์ ๋ํด ๋ฐฐ์ ์ต๋๋ค :) ๋ค์ ์์
๊น์ง!
๋ ์ฝ์ด๋ณด๊ธฐ: |
---|
GO TO FULL VERSION