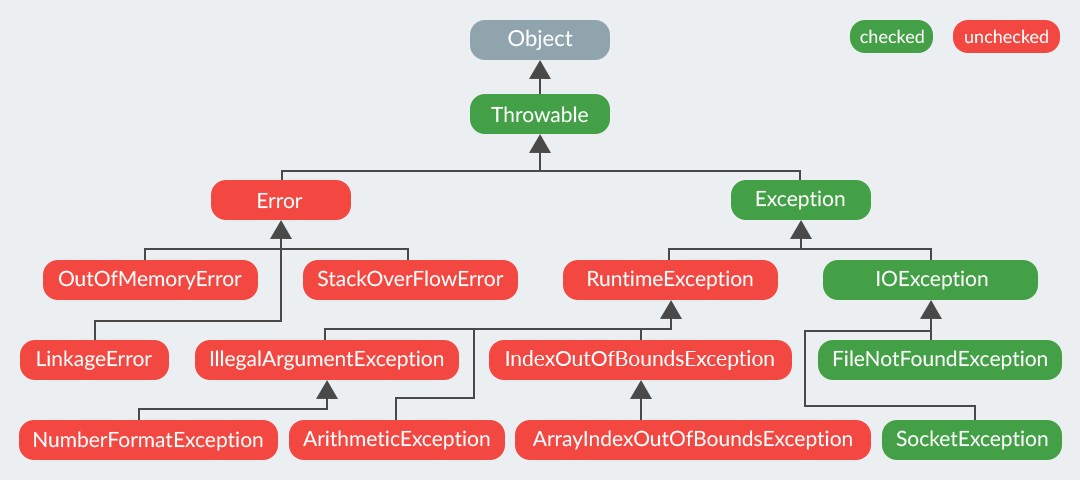
"오늘 한 가지 더 얘기하고 싶었습니다. 자바에서 모든 예외는 체크( checked ) 와 언체크 (잡아야 하는 것과 잡지 않아도 되는 것)의 두 가지 유형으로 나뉩니다. 기본적으로 모든 예외 는 잡았다."
"코드에서 의도적으로 예외를 던질 수 있습니까?"
"당신은 당신 자신의 코드에서 예외를 던질 수 있습니다. 당신은 당신 자신의 예외를 작성할 수도 있습니다. 그러나 그것에 대해서는 나중에 이야기할 것입니다. 지금 당장은 Java Machine이 던진 예외에 집중합시다."
"좋아요."
" 메서드에서 ClassNotFoundException 또는 FileNotFoundException 이 발생(발생) 하는 경우 개발자는 메서드 선언에 이를 표시해야 합니다. 이들은 확인된 예외입니다. 일반적으로 다음과 같이 표시됩니다."
확인된 예외의 예 |
---|
|
|
|
"그래서 우리는 'throws' 다음에 쉼표로 구분된 예외 목록을 씁니다. 맞죠?"
"예. 하지만 더 있습니다. 프로그램을 컴파일하려면 method1
아래 예제에서 호출하는 메서드가 두 가지 작업 중 하나를 수행해야 합니다. 이러한 예외를 포착하거나 (호출자에게) 다시 throw하여 선언에서 다시 throw된 예외를 나타냅니다. "
"다시 말하지만, 기본 메서드가 선언에 ' throws FileNotFoundException , …'가 포함된 메서드를 호출해야 하는 경우 다음 두 가지 중 하나를 수행해야 합니다.
1) FileNotFoundException 캐치 , …
안전하지 않은 메서드를 호출하는 코드를 try-catch 블록으로 래핑해야 합니다.
2) FileNotFoundException을 잡지 마십시오 , …
이러한 예외를 기본 메서드 의 throws 목록 에 추가해야 합니다 ."
"예를 들어 주시겠습니까?"
"이것 좀 보세요:"
public static void main(String[] args)
{
method1();
}
public static void method1() throws FileNotFoundException, ClassNotFoundException
{
//Throws FileNotFoundException if the file doesn't exist
FileInputStream fis = new FileInputStream("C2:\badFileName.txt");
}
"이 예제의 코드는 메인 메서드가 catch해야 하는 예외를 throw하는 method1() 을 호출 하기 때문에 컴파일되지 않습니다 ."
"컴파일하려면 기본 메소드에 예외 처리를 추가해야 합니다. 다음 두 가지 방법 중 하나로 이를 수행할 수 있습니다."
public static void main(String[] args) throws FileNotFoundException, ClassNotFoundException
{
method1();
}
public static void method1() throws FileNotFoundException, ClassNotFoundException
{
//Throws FileNotFoundException if the file doesn't exist
FileInputStream fis = new FileInputStream("C2:\badFileName.txt");
}
"여기서 try-catch 로 잡습니다 ."
public static void main(String[] args)
{
try
{
method1();
}
catch(Exception e)
{
}
}
public static void method1() throws FileNotFoundException, ClassNotFoundException
{
//Throws FileNotFoundException if the file doesn't exist
FileInputStream fis = new FileInputStream("C2:\badFileName.txt");
}
"명확해지기 시작했습니다."
"다음 예를 보십시오. 나머지를 이해하는 데 도움이 될 것입니다."
public static void method2() throws FileNotFoundException, ClassNotFoundException
{
method1();
}
public static void method3() throws ClassNotFoundException
{
try
{
method1();
}
catch (FileNotFoundException e)
{
System.out.println("FileNotFoundException has been caught.");
}
}
public static void method4()
{
try
{
method1();
}
catch (FileNotFoundException e)
{
System.out.println("FileNotFoundException has been caught.");
}
catch (ClassNotFoundException e)
{
System.out.println("ClassNotFoundException has been caught.");
}
}
"예외 유형이 하나 더 있는데 RuntimeException 과 이를 상속하는 클래스입니다. 이를 잡을 필요는 없습니다. 이들은 확인되지 않은 예외입니다. 예측하기 어려운 것으로 간주됩니다. 동일한 방식으로 처리할 수 있지만 throws 절 에 표시할 필요가 없습니다 ."