1. Comparando strings
Está tudo bem e bom. Mas você pode ver que as strings s1
e s2
são na verdade as mesmas, o que significa que elas contêm o mesmo texto. Ao comparar strings, como você diz ao programa para olhar não para os endereços dos String
objetos, mas para o seu conteúdo?
Para nos ajudar com isso, a classe Java String
possui o equals
método. Chamando fica assim:
string1.equals(string2)
Este método retorna true
se as strings forem iguais e false
se não forem iguais.
Exemplo:
Código | Observação |
---|---|
|
|
Mais exemplos:
Código | Explicação |
---|---|
|
false |
|
true |
|
true |
|
true |
2. Comparação de strings sem distinção entre maiúsculas e minúsculas
No último exemplo, você viu que a comparação produz . De fato, as cordas não são iguais. Mas..."Hello".equals("HELLO")
false
Claramente, as strings não são iguais. Dito isso, seu conteúdo tem as mesmas letras e difere apenas no caso das letras. Existe alguma maneira de compará-los e desconsiderar o caso das letras? Ou seja, então isso rende ?"Hello".equals("HELLO")
true
E a resposta a esta pergunta é sim. Em Java, o String
tipo possui outro método especial: equalsIgnoreCase
. Chamando fica assim:
string1.equalsIgnoreCase(string2)
O nome do método é traduzido aproximadamente como comparar, mas ignorar maiúsculas e minúsculas . As letras no nome do método incluem duas linhas verticais: a primeira é minúscula L
e a segunda é maiúscula i
. Não deixe que isso o confunda.
Exemplo:
Código | Observação |
---|---|
|
|
3. Exemplo de comparação de strings
Vamos dar apenas um exemplo simples: suponha que você precise inserir duas linhas do teclado e determinar se elas são iguais. É assim que o código ficará:
Scanner console = new Scanner(System.in);
String a = console.nextLine();
String b = console.nextLine();
String result = a.equals(b) ? "Same" : "Different";
System.out.println(result);
4. Uma nuance interessante de comparação de strings
Há uma nuance importante da qual você precisa estar ciente.
Se o compilador Java encontrar várias strings idênticas em seu código (especificamente em seu código), ele criará apenas um único objeto para elas para economizar memória.
String text = "This is a very important message";
String message = "This is a very important message";
E aqui está o que a memória conterá como resultado:
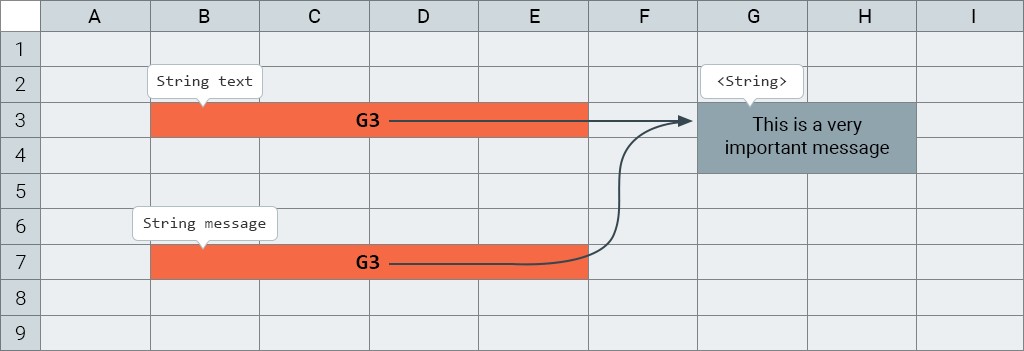
E se você comparar text == message
aqui, obterá true
. Portanto, não se surpreenda com isso.
Se, por algum motivo, você realmente precisar que as referências sejam diferentes, poderá escrever o seguinte:
String text = "This is a very important message";
String message = new String ("This is a very important message");
Ou isto:
String text = "This is a very important message";
String message = new String (text);
Em ambos os casos, as variáveis text
e message
apontam para objetos diferentes que contêm o mesmo texto.