1. 文字列の比較
これは全く問題ありません。s1
しかし、と の文字列は実際には同じであることがわかりますs2
。これは、それらに同じテキストが含まれていることを意味します。String
文字列を比較するときに、オブジェクトのアドレスではなくその内容を調べるようにプログラムに指示するにはどうすればよいでしょうか?
これを支援するために、Java のString
クラスにはequals
メソッドがあります。呼び出すと次のようになります。
string1.equals(string2)
このメソッドは、true
文字列が同じであるかどうかを返します。また、false
同じでない場合も返します。
例:
コード | ノート |
---|---|
|
|
その他の例:
コード | 説明 |
---|---|
|
false |
|
true |
|
true |
|
true |
2. 大文字と小文字を区別しない文字列比較
最後の例では、比較によりが得られることがわかりました。確かに、文字列は等しくありません。しかし..."Hello".equals("HELLO")
false
明らかに、文字列は等しくありません。とはいえ、内容は同じ文字であり、大文字と小文字が異なるだけです。大文字と小文字を無視してそれらを比較する方法はありますか? つまり、次の結果が得られますか?"Hello".equals("HELLO")
true
そして、この質問に対する答えは「はい」です。Java では、String
型には別の特別なメソッドがありますequalsIgnoreCase
。呼び出すと次のようになります。
string1.equalsIgnoreCase(string2)
このメソッドの名前は、大まかに訳すと、「比較するが、ケースを無視する」となります。メソッド名の文字には 2 つの垂直線が含まれています。1 つ目は小文字でL
、2 つ目は大文字ですi
。混乱しないでください。
例:
コード | ノート |
---|---|
|
|
3. 文字列比較の例
簡単な例を 1 つだけ挙げてみましょう。キーボードから 2 行を入力し、それらが同じかどうかを判断する必要があるとします。コードは次のようになります。
Scanner console = new Scanner(System.in);
String a = console.nextLine();
String b = console.nextLine();
String result = a.equals(b) ? "Same" : "Different";
System.out.println(result);
4. 文字列比較の興味深いニュアンス
注意すべき重要なニュアンスが 1 つあります。
Java コンパイラがコード内 (特にコード内) で複数の同一の文字列を見つけた場合、メモリを節約するために、それらに対して 1 つのオブジェクトのみを作成します。
String text = "This is a very important message";
String message = "This is a very important message";
結果としてメモリに含まれる内容は次のとおりです。
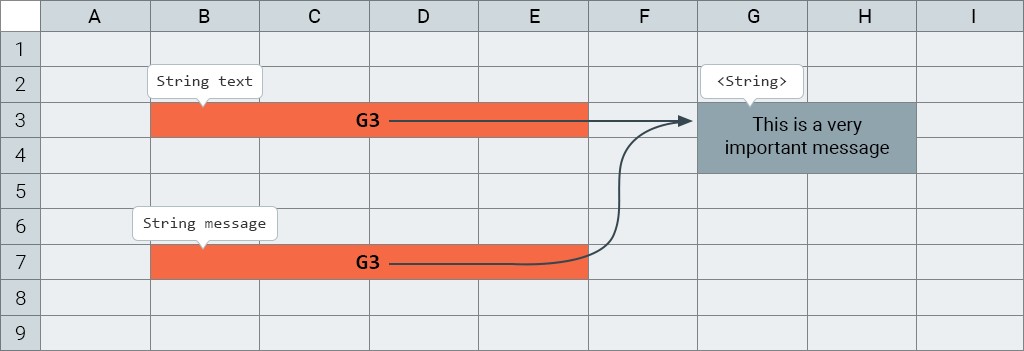
ここで比較するとtext == message
、 が得られますtrue
。だから驚かないでください。
何らかの理由で参照を実際に異なるものにする必要がある場合は、次のように記述できます。
String text = "This is a very important message";
String message = new String ("This is a very important message");
またはこれ:
String text = "This is a very important message";
String message = new String (text);
どちらの場合も、text
およびmessage
変数は、同じテキストを含む異なるオブジェクトを指します。