1. 문자열 비교
이것은 모두 훌륭하고 좋습니다. s1
그러나 및 s2
문자열이 실제로 동일하다는 것을 알 수 있습니다 . 즉, 동일한 텍스트를 포함한다는 의미입니다. 문자열을 비교할 때 객체의 주소가 아니라 String
내용을 보도록 프로그램에 지시하는 방법은 무엇입니까?
이를 돕기 위해 Java의 String
클래스 에는 equals
메서드가 있습니다. 호출하면 다음과 같습니다.
string1.equals(string2)
이 메서드는 true
문자열이 동일한 경우와 false
동일하지 않은 경우를 반환합니다.
예:
암호 | 메모 |
---|---|
|
|
더 많은 예:
암호 | 설명 |
---|---|
|
false |
|
true |
|
true |
|
true |
2. 대소문자를 구분하지 않는 문자열 비교
마지막 예에서 비교 결과가 임을 확인했습니다 . 실제로 문자열은 동일하지 않습니다. 하지만..."Hello".equals("HELLO")
false
분명히 문자열은 같지 않습니다. 즉, 내용은 동일한 문자를 가지며 문자의 대/소문자만 다릅니다. 그것들을 비교하고 문자의 대소 문자를 무시할 수있는 방법이 있습니까? 즉, 그래서 그것은 수율 ?"Hello".equals("HELLO")
true
그리고 이 질문에 대한 대답은 '예'입니다. Java에서 String
유형에는 또 다른 특수 메소드가 있습니다 equalsIgnoreCase
. 호출하면 다음과 같습니다.
string1.equalsIgnoreCase(string2)
메서드의 이름은 대략적으로 compare but ignore case 로 번역됩니다 . 메서드 이름의 문자에는 두 개의 수직선이 포함됩니다. 첫 번째는 소문자 L
이고 두 번째는 대문자입니다 i
. 그것이 당신을 혼란스럽게 하지 마십시오.
예:
암호 | 메모 |
---|---|
|
|
3. 문자열 비교의 예
한 가지 간단한 예를 들어 보겠습니다. 키보드에서 두 줄을 입력하고 같은지 여부를 확인해야 한다고 가정합니다. 코드는 다음과 같습니다.
Scanner console = new Scanner(System.in);
String a = console.nextLine();
String b = console.nextLine();
String result = a.equals(b) ? "Same" : "Different";
System.out.println(result);
4. 문자열 비교의 흥미로운 뉘앙스
알아야 할 중요한 뉘앙스가 하나 있습니다.
Java 컴파일러가 코드에서(특히 코드에서) 동일한 문자열을 여러 개 발견하면 메모리를 절약하기 위해 단일 객체만 생성합니다.
String text = "This is a very important message";
String message = "This is a very important message";
결과적으로 메모리에 포함되는 내용은 다음과 같습니다.
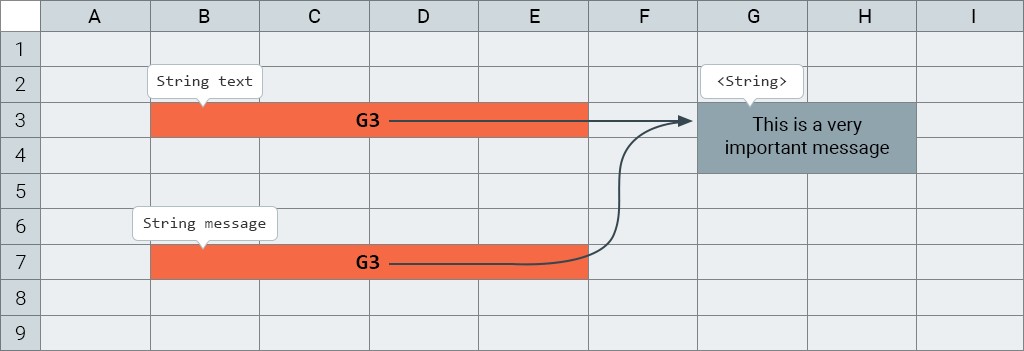
text == message
그리고 여기서 비교하면 true
. 그러니 놀라지 마세요.
어떤 이유로 참조가 실제로 달라야 하는 경우 다음과 같이 작성할 수 있습니다.
String text = "This is a very important message";
String message = new String ("This is a very important message");
아니면 이거:
String text = "This is a very important message";
String message = new String (text);
이 두 경우 모두 text
및 message
변수는 동일한 텍스트를 포함하는 다른 개체를 가리킵니다.