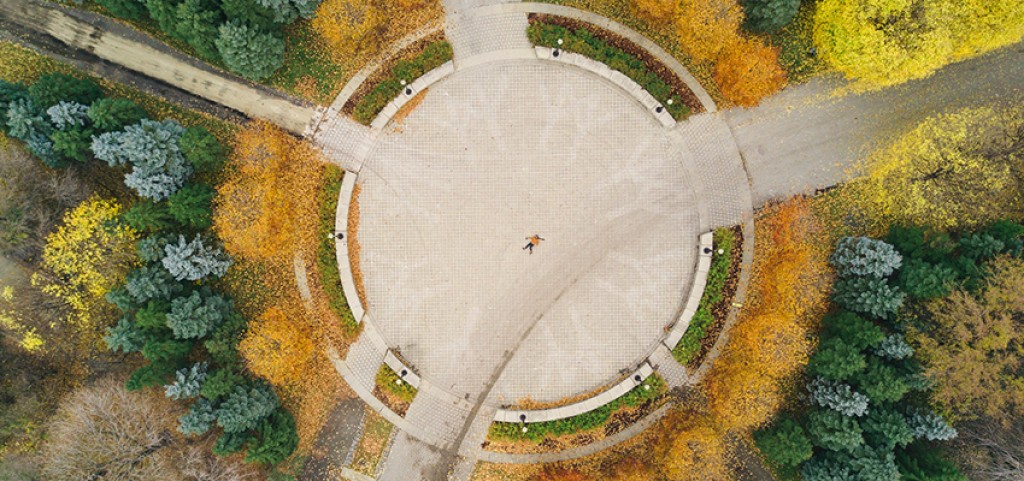
Entry threshold: High, low, medium
Programmers often talk about the "entry threshold" — a concept that reflects the amount of effort required for any given "junior developer" to master a programming language sufficiently well to write his or her first serious program and find a job. The "entry threshold" consists of knowledge of:- syntax peculiarities and nuances of the language
- libraries
- algorithms and data structures.
Web or not web?
Web
Web programmers can be divided into Frontend and Backend developers. You should understand what these terms mean. Frontend developers are involved in the client side, i.e. what the user sees. The "backend" is about manipulating and storing data — the part of a service that runs on a server. For a Frontend developer deciding which programming language to learn, JavaScript and JavaScript frameworks (Angular JS, React and others) are essential. JS dialects, such as CoffeeScript and TypeScript, are not as popular as their parent, but they can also be useful. There is also a Flash AS, and there used to be JScript and VBScript, but only dinosaurs remember this =) Besides all this, you need to understand HTML and CSS.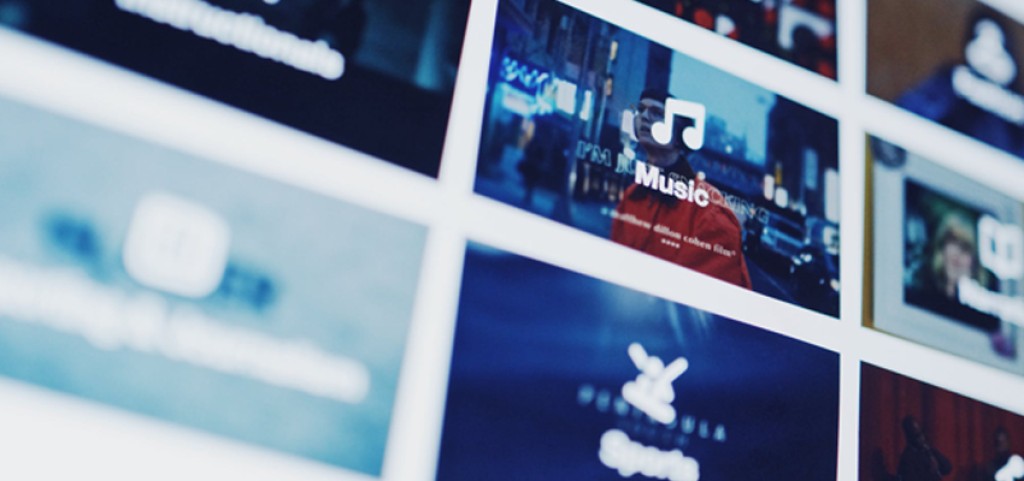
Not web (enterprise, desktop, mobile)
I've intentionally combined the following programming languages into this category with a strange name. You can use most of them to write enterprise, desktop, and even mobile applications. Python is an easy-to-understand object-oriented programming language and has become incredibly popular recently due to the growth machine learning (ML): ML developers make extensive use of Python. ML is a fairly new area in IT, and although we have already seen it bear fruit, I would not rush into this industry when choosing a programming language. First, you'll need an EXCELLENT understanding of mathematics. Second, the wave of popularity may pass in the same way as it did for "blockchain" or "nanotechnology". That said, you may recall that Python is used in web development. C++: a classic language where everything is built on the "plus-plus" operator. This language is the ancestor of all the popular object-oriented programming languages, and a beginner should definitely pay attention to it. Many popular applications have been written using it. But the excellent chance of "shooting yourself in the foot" and the difficult-to-understand syntax bring to zero the probability that a beginner will master this mastodon of programming. Kotlin, which is like Java for hipsters, is a crazy mix of OOP and functional programming. It has been popular recently due to the fact that an experienced developer switching from Java to Kotlin can seriously improve his or her productivity. An experienced developer will quickly get comfortable in this programming language. By the way, the same thing applies to Scala, but Kotlin is popular in the Android world. Java is easy for beginners to learn. Especially with the help of CodeGym =) Java syntax is understandable and though there is a risk of "shooting yourself in the foot", it is not critical.OOP or POP?
The procedural approach
The procedure-oriented approach involves writing a program consisting of sequential statements that can be assembled into a unified whole to effectively solve a specific set of problems. Such languages include C, PureBasic and Pascal. In other words, the languages that bring despair to high school students and undergraduates. There is also the relatively young GO language. That said, being familiar with procedural languages is very useful for a potential developer. My immersion in procedural languages came with the Wolfram Mathematica system and university research. Proper algorithms and simple procedures, moving linearly from the beginning of the program to the end, allowed me to calculate values relevant to modern theoretical physics. This "sequential" programming language is just the thing to help you understand that sometimes it is easier to write code that perform calculations manually. Learning procedure-oriented programming (POP) provide good algorithmic training, which an employer almost always wants to see in a job candidate. Absolutely everything in IT is built on the foundation of procedural languages, so don't underestimate them. By the way, beginners deciding which programming language to learn often think that only OOP languages support multithreading. This is not true. Procedural programming languages also allow parallel computations.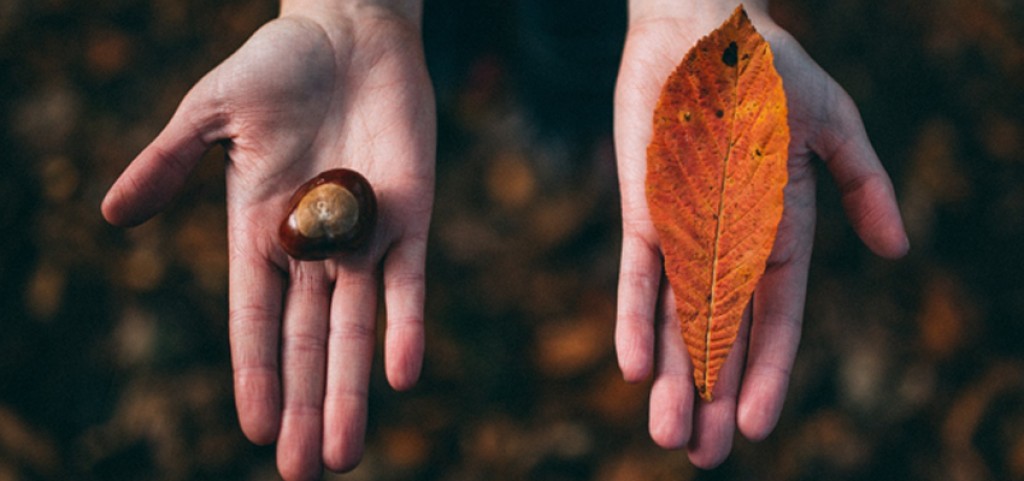
The object-oriented approach
Those who started with procedural languages are usually well versed in mathematics, algorithms, and data structures (due to technical universities' emphasis on these areas). Still, the reality today is that successful programmers are usually those who have mastered a different approach to programming: the object-oriented paradigm. The OOP ideology lets you build truly global systems. A feature of this approach is its similarity with the real world:- Different objects interact with each other and exist independently of each other.
- Objects have a hierarchy and can adopt or change the behavior of their ancestors.
- You can use abstract concepts, but only objects that actually exist can interact.
Example Procedure-oriented languages are tools for solving specific problems. If your task changes, even slightly, you'll probably have to spend time and effort rewriting all the algorithms. Imagine a program that describes a car dealership that sells cars and trucks, both new and used. In a procedural language, you need to define functions that process the input or output of data for each entity: a new car, a new truck, a used car, and a used truck. What does OOP offer? With an object-oriented approach, we just need to define a Vehicle base class that stores the characteristics shared by all vehicle types:
And methods for taking in and sending out information. Then we create objects that inherit the characteristics of the Vehicle class: Car and Truck. They contain information that pertains specifically to these types of vehicle, as well as input/output methods. Suddenly, the dealership's management decides to expand the lineup by also offering motorcycles. Under the procedural approach, we would have to recreate all the logic for new and used motorcycles, while an OOP language lets us simply create a new Motorcycle class that inherits all the characteristics of the Vehicle superclass and contains motorcycle-specific refinements. And what would happen if we add various vehicles? A procedural implementation would require more work than OOP. What's more, the larger the lineup, the fewer operations involving objects will be required. |
- OOP involves the independent development of individual modules, allowing a programmer or team to choose the method and boundaries of contact and information exchange.
- Code partitioned into small modules is much easier to read than monolithic procedures. As a result, an outsider can quickly understand your code, and likewise, you can join a new project if necessary.
- One class can be changed without affecting the interaction of another, but such a change can affect the hierarchy of child objects. Once you've mastered this approach, expanding and modifying a program become trivial.
Cross-platform.
Java works everywhere thanks to the Java virtual machine (JVM). One of this language's main advantages is its cross-platform nature: no need to think about which library to add or the architecture of a particular processor. "Write once, run anywhere."
Documentation.
There is an enormous documentation base: official Oracle documentation, training portals, and a constantly evolving community. Answers to most of the questions that arise during development can be found in a few minutes. The main thing is to understand what to enter into the search engine =)
Popularity.
Java is the most popular programming language in the world: in addition to the aforementioned Android and web developers, almost every enterprise developer writes in Java. Enterprise refers to the internal corporate development necessary for the needs of large corporations.
Every year, haters predict "the death of Java". They say, "Oracle will stop supporting it. You're totally wasting your time." This is not true! They promise to release new versions of Java every six months.
For me, the lambda expressions in Java 8 were revolutionary and a revelation, to say nothing of the new versions! I'm currently working on a "legacy" project, so I don't delve into the latest innovations, but it's a fact that Java is alive.
Android.
For the past 4 years, Android has consistently held more than 80% of the mobile phone market. TVs, media players, and even car infotainment systems run on this operating system. And app development for this OS happens mainly in Java. Just imagine the prospects that are opening up. When I got a job as an Android developer, I wondered how much the product I was developing was worth? As it turned out, the price was about $5 per year. That begs the question, "then where does the money come from for this office, the salaries, snack room, ping-pong table, robots, and other perks? The answer lies in the volume: our app has 20 million users.
Salaries.
And now the icing on the cake: a Java developer's salary is among the highest in the industry. After all, you're planning to study programming for a specific purpose: to get a good job.
The programming language's popularity
There are official sources of information, so let's turn to them. According to TIOBE, Java ranks first as of October 2019. In the PYPL ranking, Java ranks second, far ahead of JS and rivaling the trendy Python.Conclusion
As a beginner contemplates choosing a programming language, this is what he or she should pay attention to:- Popularity (Java consistently occupies a leading position)
- Entry threshold (for Java, it's medium: employers require a rather broad range of skills)
- Available materials (welcome to CodeGym =))
- Fields of application: the more fields where a programming language is used, the more specialists are required in the market. I've already mentioned how Java supports cross-platform development, but I never tire of repeating it.