What is Java Syntax?
Java Syntax is a basic of the language, all the main rules, commands, constructions to write programs that the compiler and computer “understands”. Every programming language has its syntax as well as human language. This article focuses on the basic syntax of the Java programming language and is intended for novice developers or those who know another programming language. Some aspects may not be clear to beginners. If so, it is best to skip them and focus on examples. As in everything else, it is better to learn a programming language cyclically, gradually coming to a deeper understanding of certain concepts. Every Java program is a bunch of objects that incorporate each other with data (variables) and behavior (functions or methods). Also the Java program is a class or a few classes. An object is an instance of a class. You can understand class as a model, for example, cookie cutters and objects like cookies. Or class as an abstract “Java programmer” and object as “Java Programmer John” or “Java Programmer Ivy”.Object in Java
Objects in Java have states and behaviors. Example: A cat has states: its name is Furr, color is red, owner is John; cat also has behavior Now Furr is sleeping. He could also purr, walk, and so on. An object is an instance of a class.Class in Java
Class is a model or template or blueprint of the object. It describes the behavior and states that the object of its type supports. For example, the Cat class has its name, color, owner; cat also has behavior such as eating, purring, walking, sleeping.Methods in Java
Methods are for describing the logics, manipulating data and executing all the actions. Every method defines behavior. A class can contain many methods. For example we can write a method sleep() for Cat class (to sleep) or purr() to purr.Instance Variables in Java
Every object has a unique set of instance variables. Object state is usually generated by the values assigned to these instance variables. For example cat’s name or age could be a variable. We are going to start with the simplest Java program. Using this example, we will understand the basic concepts of Java syntax, and then take a closer look at them.Simple Java program: Hello, Java!
Here's a simple Java program:
class HelloJava {
public static void main(String[] args) {
System.out.println("Hello, Java!");
}
}
This program prints out a string “Hello, Java!” to console.
I recommend you to install JDK and IntelliJ IDEA and try to write out the code you see above. Or for the first try find an online IDE to do the same.
Now let's take this program line by line, but omit some details that are not necessary for a beginner.
class HelloJava
Each program in Java is a class or more often many classes. The line class HelloJava means that here we create a new class and its name is HelloJava. As we defined above, class is a kind of template or blueprint, it describes the behavior and states of the class’ objects. It could be tough for novice programmers, you’ll learn this concept a little bit later. For now class HelloJava is just the beginning of your program.
You may have noticed the curly brace { on the same line and throughout the text. A pair of curly braces {} denotes a block, a group of programming statements that is treated as one single unit. Where { means the beginning of the unit and } its ending. Blocks can be nested within each other, or they can be sequential.
There are two nested blocks in the above program. The external one contains the body of the class Hello. The inner block contains the body of the main() method.
public static void main (String args []) {
Here is the beginning of the main method. A method is a behaviour, or the sequence of commands that allows you to perform an operation in a program. For example multiply 2 numbers or print out a string. In other words, a method is a function. In some other programming languages, methods are often referred to as "functions". Methods, just like all elements of a Java program, are located within a class. Each class can have one, many, or no methods.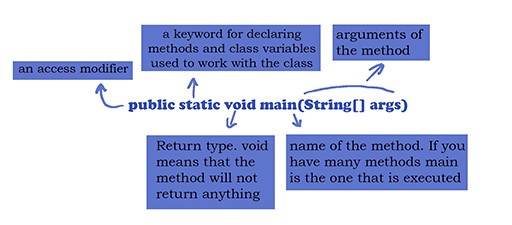
System.out.println("Hello, Java!");
Formally this line executes the println method of the out object. The out object is declared in the OutputStream class and statically initialized in the System class. However it’s a bit complicated for a total newbie. It is enough for a beginner to know that this line prints words "Hello, Java!" to the console.
So if you run the program in your IDE, you’ll get the output in console: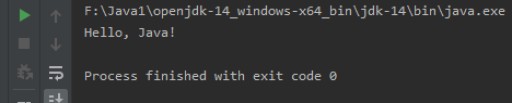
Java basic syntax rules
There are some main syntax rules to follow when programming in Java:- the file name must be identical to the class name;
- most often each class is in a separate file with a .java extension. Class files are usually grouped into folders. These folders are called packages;
- characters are case sensitive. String is not equal to string;
- The beginning of Java program processing always starts in the main method: public static void main (String [] args). The main () method is a required part of any Java program;
- Method (procedure, function) is a sequence of commands. Methods define the behavior of on object;
- The order of the methods in the program file is irrelevant;
- Keep in mind that the first letter of a class name is in uppercase. If you are using multiple words, use uppercase for the first letter of each word (“MyFirstJavaClass”);
- the names of all methods in Java syntax begin with a lowercase letter. When using multiple words, the subsequent letters are capitalized ("public void myFirstMethodName ()");
- files are saved with class name and .java extension ("MyFirstJavaClass.java");
- In Java syntax, there are delimiters "{...}" that denote a block of code and a new area of code;
- Each code statement must end with a semicolon.
- Integers: byte, short, int, long
- Fractionals: float and double
- Logical values: boolean
- Symbolic values (for representing letters and numerals): char
Java variables example:
int s;
s = 5;
char myChar = ‘a’;
In this code we created an integer variable s (an empty container) and then put a value 5 in it.
The same story with a variable named myChar. We created it with a char data type and defined it as a letter a. In this case we created a variable and simultaneously assigned a value into it. Java syntax lets you do it this way.
Reference types are some objects that keep references to values or other objects. They can also contain reference to the null. Null is a special value to denote the absence of value.
Among reference types are String, Arrays and every Class you want. If you have a Violin class, you can create a variable of this Class.
Java reference type variables example:
String s = “my words”;
Violin myViolin;
You will learn more about them later. Remember that non-primitive types of variables start from capital letters while primitive — from lowercase letters.
Example:
int i = 25;
String s = “Hello, Java!”;
Java Arrays
Arrays are objects that store multiple variables of the same type. However, an array itself is an object on the heap. We will look into how to declare, construct, and initialize in the upcoming chapters. Array example:
int[] myArray = {1,7,5};
Here we have an array that contains from the three integers (1,7 and 5)Java Enums
In addition to primitive data types Java has such a type as enum or enumeration. Enumerations represent a collection of logically related constants. An enumeration is declared using the enum operator, followed by the name of the enumeration. Then comes a comma-separated list of enumeration elements:
enum DayOfWeek {
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY,
SUNDAY
}
An enumeration actually represents a new type, so we can define a variable of that type and use it. Here is an example of using enumeration.Java Enum Example
public class MyNum{
public static void main(String[] args) {
Day myDay = DayOfWeek.FRIDAY;
System.out.println(myDay); //print a day from the enum
}
}
enum DayOfWeek{
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY,
SUNDAY
}
If you run the program, FRIDAY is printed in the console. You can put your Enum and MyNum class code in one file, but it is better to create two separate files: one for MyNum class and one for Day enum. IntelliJ IDEA lets you choose enum while creating.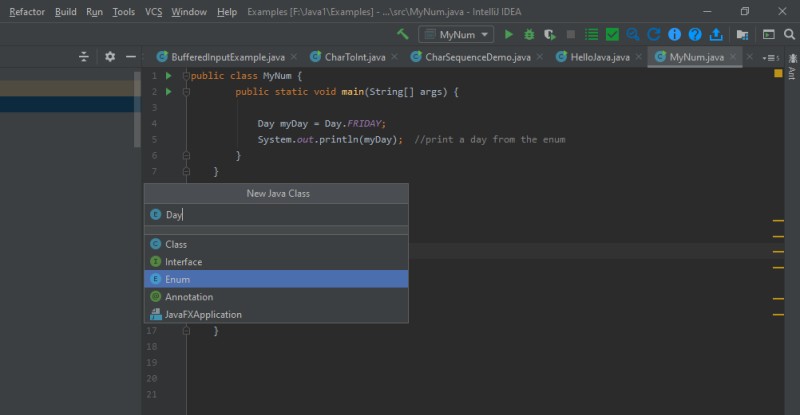
Declaring Variables in Java
Actually we have declared some variables above and even identified them. Declaration is a process of allocating memory for a variable of a certain type and naming it. Something like that:
int i;
boolean boo;
We can also declare to initialize a variable using assignment operator (=). That means we put a particular value into the memory we allocated. We can do it right in a moment of declaration or later.declaring a variable example
String str;
int i = 5;
Str = “here is my string”;
If you declare a variable without initialization it gets some default value anyway. For int this value is 0, for String or any other reference type it is a special null identifier.Java Identifiers
Identifiers are just names of Java components — classes, variables, and methods. All Java components should have names.
Class Violin {
int age;
String masterName;
}
Violin is the class identifier.
age and masterName are variables identifiers.
Here some Java identifiers rules:- All identifiers begin with a latin letter (A to Z or a to z), currency character ($) or an underscore (_).
- After the first character, identifiers can have any combination of characters.
- A Java keyword can’t be an identifier (you find out of keywords a little bit later).
- Identifiers are case sensitive.
Identifiers example
Legal identifiers: java, $mySalary, _something Illegal identifiers: 1stPart, -oneJava Modifiers
Modifiers are special words of the Java language you can use to modify elements (classes, methods, variables). Java hase two categories of modifiers: access and non-access Modifiers.Access modifiers example
There are 4 access modifiers in Java:- public. A public element It can be accessed from the class, outside the class, inside and outside the package
- Element with default (empty) modifier can be accessed only within the package
- protected modifier can be accessed inside and outside the package through child class
- private element available only within the class it declared.
Non-access modifiers example
There are 7 of them- static
- final
- abstract
- synchronized
- transient
- volatile
- native
Java Keywords
Java Keywords are the special words to use in Java that act as a key to a code. They are also well known as reserved words: you can’t use them for identifiers of variables, methods, classes, etc. Here they are:- abstract: keyword to declare abstract class.
- boolean: Java boolean keyword to declare a variable as a boolean type. Such variables can be only true and false.
- break: use Java break keyword to break loop or switch statement.
- byte: Java byte keyword for declaration a one byte whole number variable.
- case: is used to with the switch statements to mark blocks of text.
- catch: is used to catch the exceptions after the try block.
- char: Java char keyword for a character variable. It can hold unsigned 16-bit Unicode characters.
- class: Java class keyword to declare a class.
- continue: Java keyword to continue the loop.
- default: Java default keyword to specify the default block of code in a switch statement.
- do: is used in do-while loop construction.
- double: Java double keyword is used to declare a number variable. It can hold 8 byte floating-point numbers.
- else: you can use it in else-if conditional statements.
- enum: is used to define a fixed set of constants.
- extends: Java extends keyword to indicate that a class extends another class (is a Child class of the other class).
- final: keyword to indicate that a variable is a constant.
- finally: marks a block of code that will be executed in spite of whether an exception is handled or not.
- float: a variable that holds a 4-byte floating-point number.
- for: a keyword to start a for loop. It is used to execute a set of instructions repeatedly while some conditions are true.
- if: keyword for checking the condition. It executes the block if the condition is true.
- implements: the keyword to implement an interface.
- import: Java import keyword to import a package, class or interface.
- instanceof: checks whether the object is an instance of a particular class or interface.
- int: a variable that can hold a 4-byte signed integer number.
- interface: Java interface keyword is used to declare an interface.
- long: a variable that can hold a 8-byte signed integer number.
- native: specifies that a method is implemented in native code using JNI (Java Native Interface).
- new: Java new keyword to create new objects.
- package: declares a Java package (folder) for files of Java classes.
- private: an access modifier indicates that a method or variable may is visible only in the class it’s declared.
- protected: an access modifier indicates that a method or variable can be accessed inside and outside the package through child class.
- public: an access modifier indicates that an element is accessible anywhere.
- return: returns a result of a method’s execution.
- short: a variable that can hold a 2-byte signed integer number.
- static: indicates that a variable or method is a class, not an object, method.
- strictfp: restricts the floating-point calculations.
- super: refers to parent class object.
- switch: selects a code block (or many of them) to be executed.
- synchronized: a non-access modifier. It specifies that method can only be accessed by one thread at a time.
- this: refers the current object in a method or constructor.
- throw: is used to explicitly throw an exception.
- throws: The declares an exception.
- transient: a transient data piece can’t be serialized.
- try: starts a block of code that will be checked for exceptions.
- void: specifies that a method doesn’t return a value.
- volatile: indicates that a variable may change asynchronously.
- while: starts a while loop. iterates a part of the program several times while the condition is true.
Comments in Java
Java supports single-line and multi-line comments. All characters available inside any comment and they are ignored by Java compiler. Developers use them to explain the code or to recall about something. Comment examples:
//single-line comment
/*here we have a multi-line comment. As you can see it uses slash and asterisks from both sides of it.*/
public class HelloJava {
/* this program was created to demonstrate comments in Java. This one is a multi-line comment.
You can use such comments anywhere in your programs*/
public static void main(String[] args) {
//here is a single-line comment
String j = "Java"; //This is my string
int a = 15; //here I have an integer
System.out.println("Hello, " + j + " " + a + "!");
int[] myArray = {1,2,5};
System.out.println(myArray.length);
}
}
Literals in Java
Literals in Java are some constant values assigned to the variable. They could be numbers or texts or something else to represent a value.- Integral literals
- Floating point literals
- Char literals
- String literals
- Boolean literals
Java literals examples
int i = 100; //100 is an integral literal
double d = 10.2;//10.2 is a floating point literal
char c = ‘b’; //b is a char literal
String myString = “Hello!”;
boolean bool = true;
Note: null is also literal.Basic operators in Java
There are different types of operators: Arithmetic- + (numbers addition and String concatenation)
- – (minus or subtraction)
- * (multiplication)
- / (division)
- % (modulus or remainder)
- < (less than)
- <= (less than or equal to)
- > (greater than)
- >= (greater than or equal to)
- == (equal to)
- != (NOT equal to)
- && (AND)
- || (OR)
- ! (NOT)
- ^ (XOR)
public class NumbersOperations {
int a;
int b;
public static int add(int a,int b){
return a+b;
}
public static int sub (int a, int b){
return a-b;
}
public static double div (double a, int b){
return a/b;
}
}
Here we have a class with tree methods to manipulate with 2 numbers. You may try to write the 4th method int mul (int a, int b) to multiply 2 numbers within this program.
Let’s also create a class to demonstrate NumberOprations work:
public class NumberOperationsDemo {
public static void main(String[] args) {
int c = NumbersOperations.add(4,5);
System.out.println(c);
double d = NumbersOperations.div(1,2);
System.out.println(d);
}
}
If you run NumberOperationsDemo, you’ll get the next output:
9
0.5
GO TO FULL VERSION