The while loop is found in almost every programming language, and Java is no exception. While loop in Java and other languages executes a statement or block of statements as long as the value of the condition that triggered it is true. In this article, we are going to talk about how to work with the while loop.
![Java While Loop - 1]()
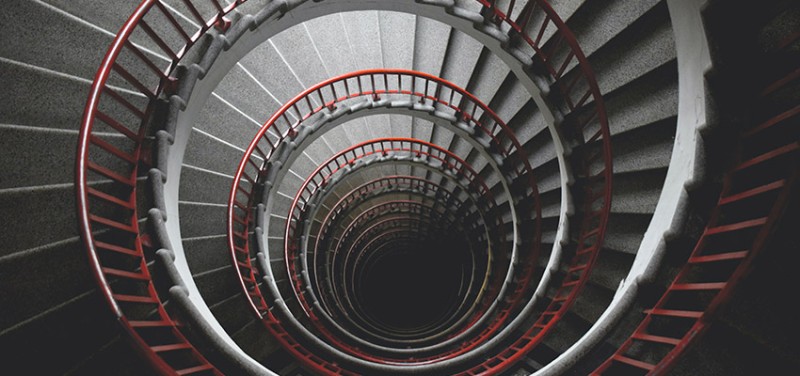
What are cycles for?
Your very first programs were a sequence of instructions that were executed step by step one after the other. Without forks and repetitions. Later we began using branches with the help of conditional operators. Well, in order to perform repetitive actions, there are cycles. Perhaps cycles are one of the most obvious benefits to novice programmers that process automation provides. Imagine that you need to write a tracker program that counts the number of steps taken in a day. And when you pass, for example, 10,000 steps, the program should send you a notification about the achieved goal. The task of issuing a notification can be expressed in words (or pseudocode) like this:While (number of steps less than 10,000)
{
Count steps
}
Send notification "you have reached the goal"
That is, as soon as the number of steps is equal to what is specified in the condition, the program exits this cycle and sends a notification. Or another example. Imagine that you need to memorize a poem (or speech). A poem is considered memorized if you can read it three times in a row without peeking. If you imagine a program that captures your attempts to recite a poem, it would also have to use a loop:
While (success < 3)
learn a poem
Similar constructions are used in all modern educational apps that are used, for example, in learning languages or playing musical instruments.
While loop and its structure
To solve problems like the examples above, and more generally, to automate repetitive actions in Java, you can use the while loop. Java while loop will work well in our case. This design arranges multiple actions in a concise and understandable structure. The while loop statement is found in almost all programming languages. It repeats a statement or block of statements as long as the value of its control expression is true. The form of the while loop is as follows:while(condition == true) {
// statement or loop body
}
The condition must be a boolean expression, that is, one that can be said to be true or false. Here are some examples of boolean expressions:
s != "exit";
a > 5;
true;
The body of the loop will be executed as long as the conditional expression is true. When the condition becomes false, control is transferred to the line of code that comes after the loop. If only one statement is used in the loop, then curly braces can be omitted (but it is better not to do this).
The logical expression is evaluated before the start of the while loop, and then each time before the next repetition of the statement is executed.
While Loop Examples
Let's write a program to sum up all the numbers from 1 to 10 using a while loop:public class WhileTest0 {
public static void main(String[] args) {
int i = 0;
int sum = 0;
//while loop
while (i < 11) {
sum = sum + i;
i++;
}
System.out.println(sum);
}
}
The output is:
55
We need to calculate the sum of all numbers from 1 (or from zero, it doesn’t matter) to 10. We make the initial sum and the first element equal to zero, and we will increase the element by one with each pass of the loop. We will continue to sum until the argument becomes equal to 10 (that is, less than 11. Similarly, we could write the condition i <= 10).
Let's take another Java while loop example. We are going to write a program where users enter positive integers. If they enter zero or a negative number, the program should report this and finish the job.
import java.util.Scanner;
public class WhileTest {
public static void main(String[] args) {
int positiveNumber = 1;
Scanner scanner = new Scanner(String.valueOf(positiveNumber));
while (positiveNumber > 0) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter positive number:");
positiveNumber = sc.nextInt();
System.out.println("ok, next");
}
System.out.println("The number should be positive");
}
}
You can improve the program and add to it, for example, checking if the user entered exactly a number and not some other character, or that the number is an integer.
do-while loop
The classic while loop, as in the examples above, immediately checks to see if the condition is true. Therefore, it can be called a cycle with a precondition. The while loop has a brother do-while, a loop with a postcondition. That is, before the test of the truth of the condition occurs, such a loop will be executed at least once:do {
// Loop body - periodically executed statement(s)
}while(Boolean expression);
So, the first example with such a loop would look like this:
public class WhileTest0 {
public static void main(String[] args) {
int i = 0;
int sum = 0;
do
{
sum = sum + i;
i++;
} while (i < 11);
System.out.println(sum);
}
}
Endless cycle
The while loop can be used to organize infinite loops in the form while(true): For example, here is a program that endlessly (with some nuances) prints a series of integers:public class EndlessLoop {
public static void main(String[] args) {
int i = 0;
while (true) {
System.out.println(i++);
}
}
}
To reinforce what you learned, we suggest you watch a video lesson from our Java Course