Iterator
Iterator is a behavioral design pattern. Represents an object that allows sequential access to the elements of an aggregate object without using descriptions of each of the aggregated objects.
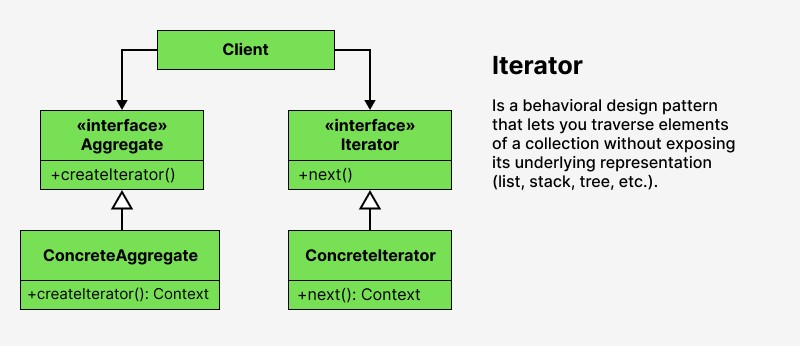
For example, elements such as a tree, a linked list, a hash table, and an array can be traversed (and modified) using an Iterator object.
Iterating through the elements is done by the iterator object, not by the collection itself. This simplifies the interface and implementation of the collection, and promotes a more logical separation of concerns.
A feature of a fully implemented iterator is that the code that uses the iterator may not know anything about the type of the iterated aggregate.
This approach is used very often. For example, you send a SQL query to the database, and in response it returns you an iterator (in SQL terms, it is usually called a cursor). And with the help of the resulting iterator, you can take rows from the SQL response one by one.
Command
A Command is a behavioral design pattern used in object-oriented programming that represents an action. The command object contains the action itself and its parameters.
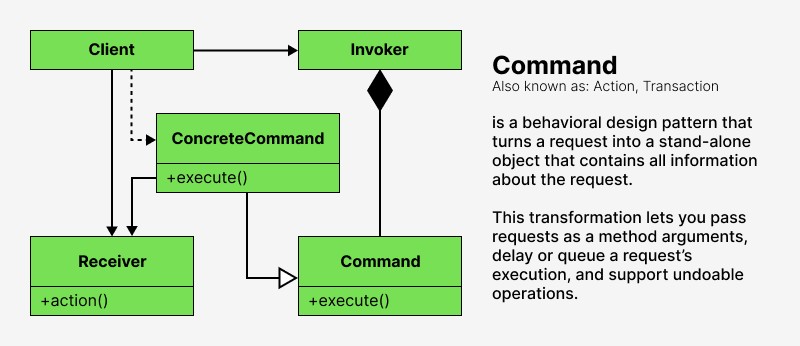
To call a method, you usually need:
- object reference
- method name (method reference)
- method parameter values
- reference to the context that contains the objects used
All this data needs to be packed into one object - Command ( command ).
But that's not all: after all, someone must execute the command. So this pattern includes four more entities: commands ( command ), a command receiver ( receiver ), a command caller ( invoker ) and a client ( client ).
An objectcommandknows about the receiver and calls the receiver method. Receiver parameter values are stored in the command. The caller (invoker) knows how to execute the command and possibly keeps track of the executed commands. The caller (invoker) knows nothing about a particular command, it only knows about the interface.
Both objects (the calling object and several command objects) belong to the client object. The client decides which commands to execute and when. To execute a command, it passes the command object to the caller (invoker).
Using command objects makes it easy to build shared components that you need to delegate or make method calls at any time without having to know the class methods or method parameters.
Using the caller object (invoker) allows you to keep track of executed commands without the need for the client to know about this accounting model (such accounting can be useful, for example, to implement undo and redo commands).
For example, you are writing a program that allows you to perform various tasks on a schedule. On the one hand, your program keeps track of tasks and manages their launch, on the other hand, it can have several executors, each of which can execute commands of its own type. For example, sending SMS, sending letters, sending messages to Telegram, etc.
Observer
Observer is a behavioral design pattern. Implements a class mechanism that allows an object of this class to receive notifications about changes in the state of other objects and thus observe them.
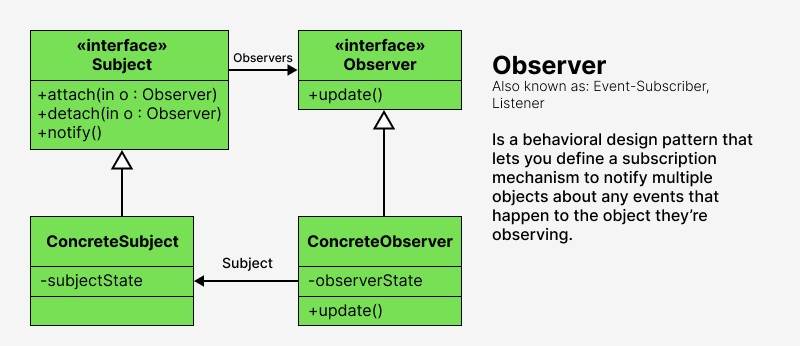
Classes that other classes subscribe to are called Subjects , and subscribing classes are called Observers .
When implementing the Observer pattern, the following classes are commonly used:
- Observable - an interface that defines methods for adding, removing and notifying observers;
- Observer - the interface through which the observer receives the notification;
- ConcreteObservable is a concrete class that implements the Observable interface ;
- ConcreteObserver is a concrete class that implements the Observer interface .
The Observer pattern is used when the system:
- there is at least one object that sends messages;
- there is at least one recipient of messages, and their number and composition may change while the application is running;
- avoids strong coupling of interacting classes.
This pattern is often used in situations in which the sender of messages is not interested in what the recipients do with the information provided to them.
Visitor
Visitor is a behavioral design pattern that describes an operation that is performed on objects of other classes. When visitingor changes, there is no need to change the serviced classes.
The template demonstrates the classic technique for recovering lost type information without resorting to double-dispatching downcast.
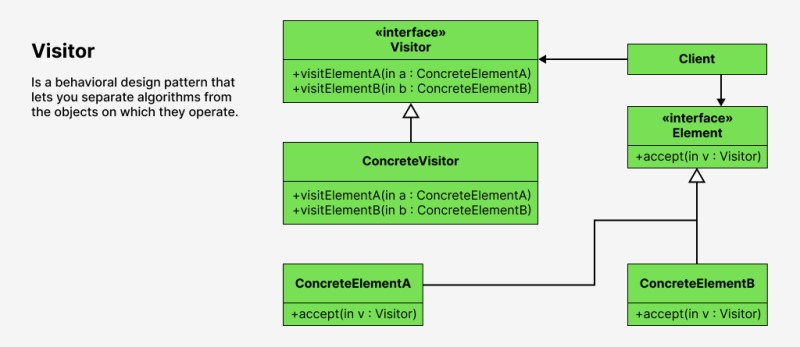
You need to do some disconnected operations on a number of objects, but you need to avoid polluting their code. And there is no way or desire to query the type of each node and cast the pointer to the correct type before performing the desired operation.
The template should be used if:
- there are various objects of different classes with different interfaces, but operations must be performed on them that depend on specific classes;
- on the structure, it is necessary to perform various operations complicating the structure;
- new operations on the structure are often added.
Mediator
Mediator is a behavioral design pattern that allows multiple objects to interact while maintaining loose coupling and avoiding the need for objects to explicitly refer to each other.
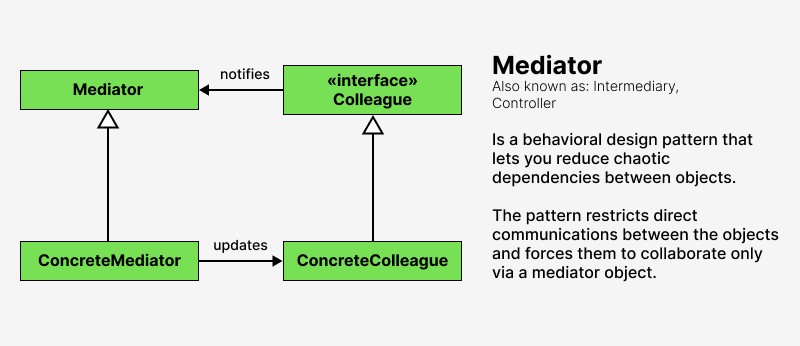
The Mediator pattern allows you to ensure the interaction of many objects, while forming a loose coupling and eliminating the need for objects to explicitly refer to each other.
The mediator defines an interface for exchanging information with objectsColleagues, A particular mediator coordinates the actions of objectsColleagues.
Each Colleague class knows about its objectMediator, all Colleagues exchange information only with an intermediary, in his absence they would have to exchange information directly.
Colleaguessend requests to the Reseller/span> and receive requests from it. The mediator implements cooperative behavior by forwarding each request to one or moreColleagues.
GO TO FULL VERSION