Recall what garbage collection is in Java
Garbage collection is the process of reclaiming full runtime memory by destroying unused objects.
Sometimes the programmer may forget to destroy useless objects, and the memory allocated to them is not freed. More and more system memory is consumed, and eventually no more is allocated. Such applications suffer from “memory leaks”.
After a certain point, there is no longer enough memory to create new objects and the program terminates abnormally due to OutOfMemoryError .
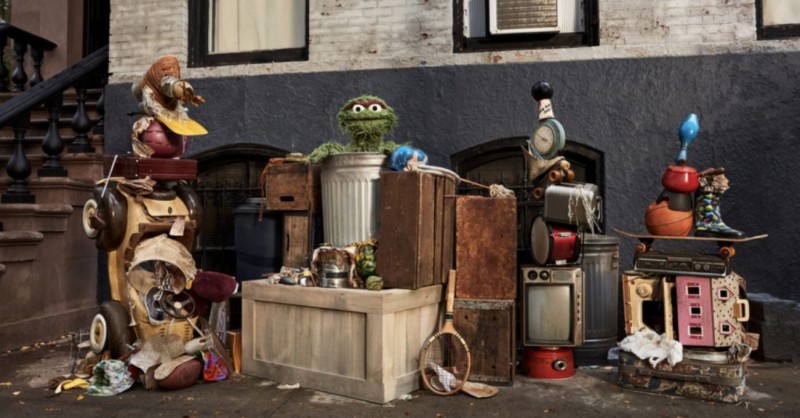
Garbage collection in Java is the process by which Java programs manage memory automatically. Java programs are compiled into bytecode that runs on the Java Virtual Machine (JVM).
When Java programs run on the JVM, objects are created on the heap, which is the portion of memory allocated to them.
While a Java application is running, new objects are created in it. In the end, some objects are no longer needed. We can say that at any given time, heap memory consists of two types of objects.
- Live - These objects are used, they are referenced from somewhere else.
- Dead - these objects are not used anywhere else, there are no references to them.
The garbage collector finds these unused objects and removes them to free up memory.
Garbage collection in Java is an automatic process . The programmer does not need to explicitly mark objects to be deleted.
Each JVM can implement its own version of garbage collection. However, the collector must conform to the standard JVM specification for dealing with objects present in heap memory to mark or identify unreachable objects and destroy them via compaction.
Object Reachability
In order to recognize an object as alive, the presence of links is not enough. This is because some dead objects can refer to other dead objects. That is why it is necessary that among all references to an object, there should be at least one from a “live” object.
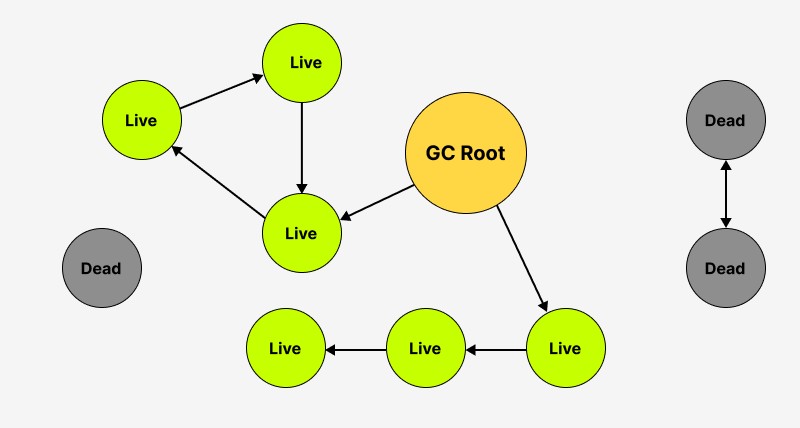
Garbage collectors work with the concept of GC Roots ( garbage collection roots ) in order to distinguish between living and dead objects. There are 100% live objects and from them there are links that animate other objects and so on.
Examples of such roots:
- Classes that are loaded by the system class loader.
- Live streams.
- Parameters of currently executing methods and local variables.
- Objects that are used as a monitor for synchronization.
- Objects that are retained from garbage collection for some purpose.
- The garbage collector walks through the entire graph of objects in memory, starting at these roots and following references to other objects.
Garbage collection steps in Java
The standard garbage collection implementation has three steps.
1. Mark objects as live
At this point, the garbage collector (GC) must identify all living objects in memory by traversing the object graph.
When it visits an object, it marks it as available and therefore alive. All objects that are not accessible from the GC roots are considered candidates for garbage collection.

2. Cleaning up dead objects
After the markup phase, the memory space is occupied by either living (visited) or dead (not visited) objects. The cleanup phase frees the memory fragments that contain these dead objects.

3. Compact arrangement of the remaining objects in memory
It is not necessary for the dead objects that were removed in the previous phase to be next to each other. Thus, you risk getting a fragmented (half-empty) memory space.
But, of course, having provided for this, it is possible to compact the memory at the moment when the garbage collector removes dead objects. The rest will be located in a contiguous block at the beginning of the heap.
The compaction process makes it easier to sequentially allocate memory for new objects.
