Executor
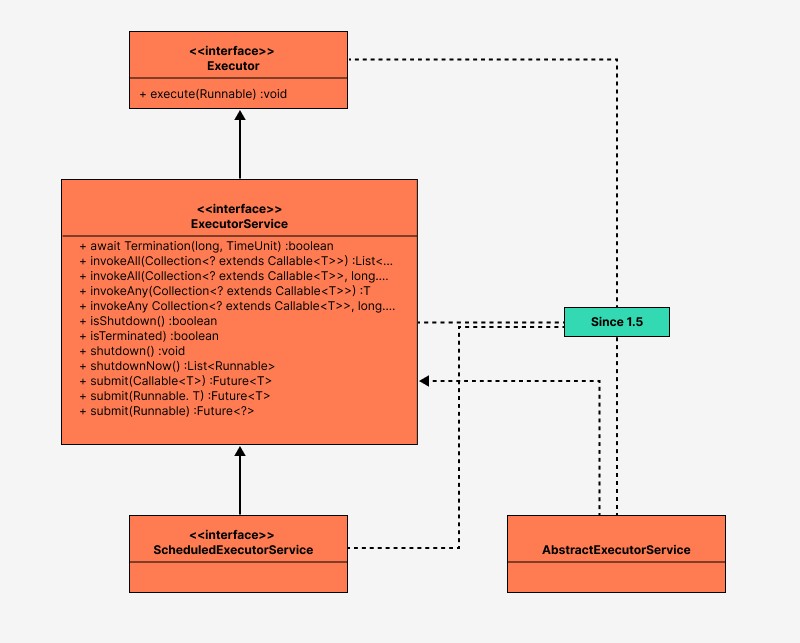
Executor is the base interface for classes that implements the launch of Runnable tasks. This provides assistance with adding a task and how to start it.
ExecutorService is an interface that extends the properties of Executor and which describes a service for running Runnable or Callable tasks. The submit methods accept a task in the form of a Callable or Runnable as an input , and a Future is used as a return value, through which you can get the result.
The invokeAll method is responsible for executing tasks, returning a list of tasks with their status and completion results.
The invokeAny method is responsible for executing tasks, returning the result of a successfully completed task (that is, without throwing an exception), if any.
ScheduledExecutorService - this interface adds the ability to run pending tasks with a certain delay or a certain period.
AbstractExecutorService is an abstract class for constructing an ExecutorService 'a. Inside there is an implementation of the submit , invokeAll , invokeAny methods . This class inherits ThreadPoolExecutor , ScheduledThreadPoolExecutor and ForkJoinPool .
public static void main(String[] args) {
ScheduledExecutorService scheduledExecutorService = Executors.newScheduledThreadPool(1);
Callable<String> task = () -> {
System.out.println(Thread.currentThread().getName());
return Thread.currentThread().getName();
};
scheduledExecutorService.schedule(task, 10, TimeUnit.SECONDS);
scheduledExecutorService.shutdown();
}
ThreadPoolExecutor
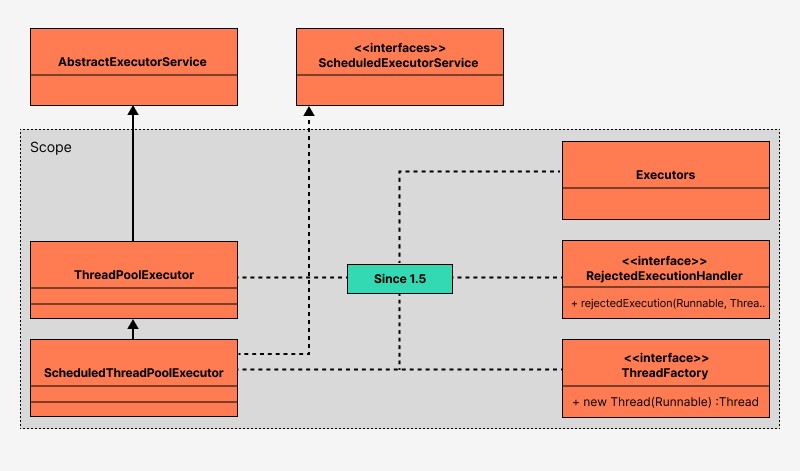
Executors is a factory class for creating ThreadPoolExecutor , ScheduledThreadPoolExecutor . If you need to create one of these pools, then this factory is exactly what you need. It contains different adapters Runnable-Callable, PrivilegedAction-Callable, PrivilegedExceptionAction-Callable and others. Has static methods for creating different ThreadPool .
ThreadPoolExecutor - Implements the Executor and ExecutorService interfaces and separates task creation from task execution. We need to implement Runnable objects and send them to the executor, and ThreadPoolExecutor is responsible for their execution, instantiation and work with threads.
ScheduledThreadPoolExecutor - In addition to the ThreadPoolExecutor methods , it creates a pool of threads that can schedule commands to execute after a given delay or for periodic execution.
ThreadFactoryis an object that creates new threads on demand. We need to pass an instance to the Executors.newSingleThreadExecutor(ThreadFactory threadFactory) method .
ExecutorService executorService = Executors.newSingleThreadExecutor(new ThreadFactory() {
@Override public Thread newThread(Runnable r) {
Thread thread = new Thread(r, "MyThread");
thread.setPriority(Thread.MAX_PRIORITY);
return thread; }
});
RejectedExecutionHandler - allows you to define a handler for tasks that for some reason cannot be executed through the ThreadPoolExecutor . This happens when there are no free streams or the service is shut down or shutdown.
Several standard implementations are found in the ThreadPoolExecutor class :
- CallerRunsPolicy - runs a task on the calling thread;
- AbortPolicy - throws an exception;
- DiscardPolicy - ignores the task;
- DiscardOldestPolicy - Removes the oldest unstarted task from the queue, then tries to add a new task again.
Completion Service
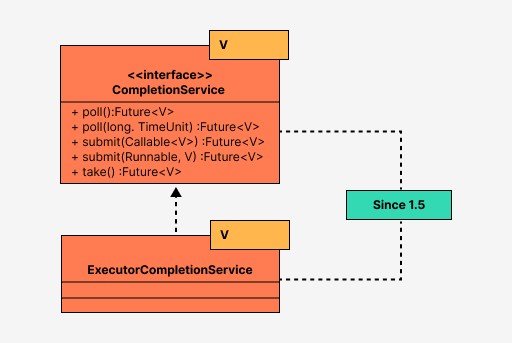
CompletionService is a service interface with decoupling of launching asynchronous tasks and getting results. To add tasks, there is a submitmethod, and to get the results of already completed tasks, a blockingtakeand a non-blockingpoll.
ExecutorCompletionService is a wrapper over any class that implements the Executor interface , such as ThreadPoolExecutor or ForkJoinPool . It is used when it is necessary to abstract from the method of launching tasks and controlling their execution.
If there are completed tasks, then we pull them out. If there are no tasks, then we hang in take until something is completed. The service uses a LinkedBlockingQueue at its core, but you can pass in any BlockingQueue implementation.
GO TO FULL VERSION