"Hello, Amigo! It's me—again. I want to give you another point of view on interfaces. You see, most of the time a class is a model of a particular object. By contrast, interfaces are more like an object's abilities or roles, rather than the object itself."
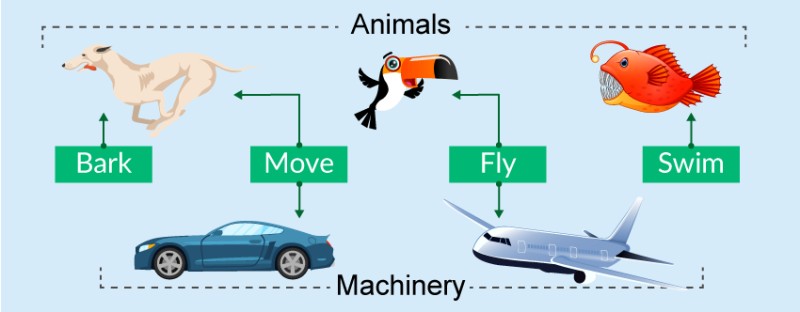
For example, things such as cars, bicycles, motorcycles, and wheels are best represented as classes and objects. But their abilities, like «I can move», «I can carry people», and «I can park», are better represented as interfaces. Check out this example:
Java code | Description |
---|---|
|
Corresponds to the ability to move. |
|
Corresponds to the ability to move. |
|
Corresponds to the ability to carry cargo. |
|
A «wheel» class. Has the ability to move. |
|
A «car» class. Has the ability to move, be driven by a person, and carry cargo. |
|
A «skateboard» class. Has the ability to move and be controlled by a person. |
Interfaces greatly simplify the life of the programmer. Programs very often have thousands of objects, hundreds of classes, and just a couple dozen interfaces (roles). There are few roles, but they can be combined in many ways (classes).
The whole point is that you don't have to write code defining interaction with every other class. All you have to do is interact with roles (interfaces).
Imagine that you're a robotic builder. You have dozens of subordinate robots and each of them can have multiple skills. Suppose you need to urgently finish building a wall. You just take all the robots that have the ability to "build" and tell them to build the wall. You don't really care which robots do it. Let it be a robotic watering can. If it knows how to build, let it build.Here's how it would look in code:
Java code | Description |
---|---|
|
Ability to «build a wall». Understands the command «build a wall» (has the appropriate method). |
|
Robots that have this ability/skill.
A watering can can't build a wall (it doesn't implement the WallBuilder interface). |
|
How do we give the command to build a wall? |
"That's amazingly interesting. I never dreamed that interfaces could be such an interesting topic."
"And then some! Together with polymorphism, it's totally mind-blowing."
GO TO FULL VERSION