"Hi, Amigo!"
"Hello, Captain Squirrels, sir!"
"You have a new secret mission today. In order to encrypt correspondence between our units, you need to implement your own URL shortener service."
"Cool! Uhh, I mean, I am ready, sir. But why do we need this?"
"What do you mean why? Soldier, we're surrounded by enemies. We need to protect our lines of communication. Proceed to the task."
"Yes, sir! Implement a URL shortener service."
"Go to our secret agent IntelliJ IDEA. You'll receive all of the instructions there."
"It shall be done, Captain!"
"Proceed."
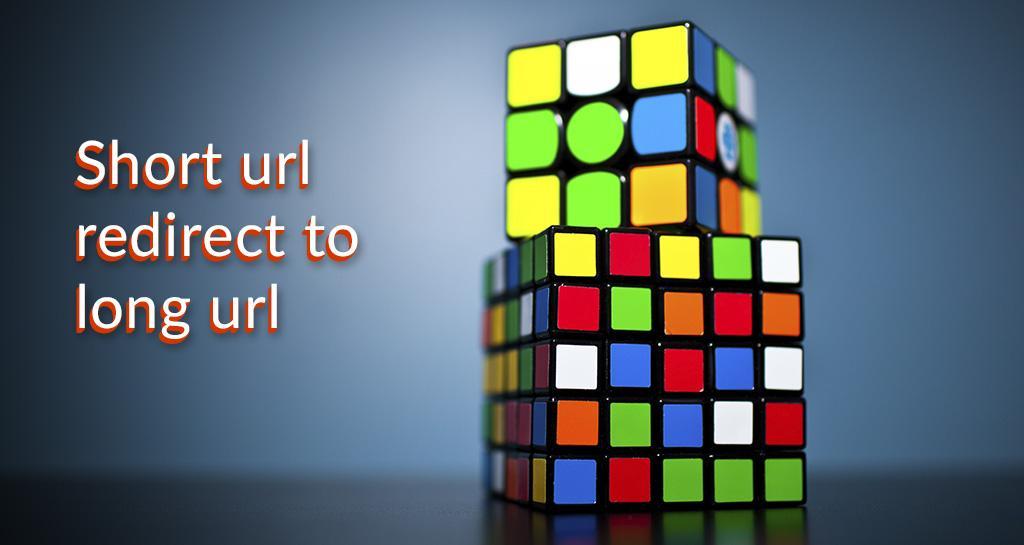
16
Task
Java Collections,
level 6,
lesson 15
Locked
Shortener (part 1)
Let's write a Shortener. It will be similar to
Google URL Shortener (https://goo.gl), but we'll extend its functionality and
make it console-based. It will shorten not only links, but also any string.
9
Task
Java Collections,
level 6,
lesson 15
Locked
Shortener (part 2)
Shortener will support various data storage strategies
(for strings and string identifiers). All these strategies will be inherited from the
StorageStrategy interface. Read more about the Strategy pattern on Wikipedia.
Our repository will rely on two concepts: key and value.
16
Task
Java Collections,
level 6,
lesson 15
Locked
Shortener (part 3)
Let's return to the Shortener class:
3.1. Add a Long lastId field to it. Initialize it to zero. This field will
hold the identifier of the last string
added to the repository.
3.2. Add a StorageStrategy storageStrategy field that will store the
data storage strategy.
16
Task
Java Collections,
level 6,
lesson 15
Locked
Shortener (4)
We'll need several helper classes:
4.1. Create a Helper class.
4.1.1. Add a static String generateRandomString() method, which
will generate a random string. Use the SecureRandom
and BigInteger classes to do this. Hint: search for "random string Java" on Google.
16
Task
Java Collections,
level 6,
lesson 15
Locked
Shortener (5)
Let's write our first repository (storage strategy). Internally, it will
have an ordinary HashMap. We'll keep all the strategies in the strategy package.
5.1. Create a HashMapStorageStrategy class that implements the StorageStrategy interface.
32
Task
Java Collections,
level 6,
lesson 15
Locked
Shortener (6)
The first strategy is ready. It's time to test it. To do this:
6.1. Create a Solution class if you haven't already done so.
6.2. Add implementations of the static helper methods to the Solution class:
6.2.1. Set<Long> getIds(Shortener shortener, Set<String> strings).
32
Task
Java Collections,
level 6,
lesson 15
Locked
Shortener (7)
Let's start implementing the second strategy: OurHashMapStorageStrategy. It won't
use the ready-made HashMap from the standard library, but rather will itself be
a collection.
7.1. Understand how the standard HashMap works. Look at its source code or
google for articles about it.
32
Task
Java Collections,
level 6,
lesson 15
Locked
Shortener (8)
Add and implement a OurHashMapStorageStrategy class, using the Entry class from the
previous subtask. The OurHashMapStorageStrategy class must implement
the StorageStrategy interface.
8.1. Add the following fields to the class:
8.1.1. static final int DEFAULT_INITIAL_CAPACITY = 16.
32
Task
Java Collections,
level 6,
lesson 15
Locked
Shortener (9)
Let's write another strategy. We'll call it FileStorageStrategy. It will be very similar
to OurHashMapStorageStrategy, but files will be
the buckets. I'm sure you know what I mean by buckets. If not, copy the internal workings
of HashMap.
9.1. Create a FileBucket class in the strategy package.
32
Task
Java Collections,
level 6,
lesson 15
Locked
Shortener (10)
Create and implement a FileStorageStrategy class. It must:
10.1. Implement the StorageStrategy interface.
10.2. Use FileBucket as a buckets. Hint: The class
must have a FileBucket[] table field.
16
Task
Java Collections,
level 6,
lesson 15
Locked
Shortener (11)
As you've noticed, getting an identifier using a string takes a lot more
time than getting a string using an identifier. This is expected and is a consequence of
HashMap's implementation. Let's write a fourth strategy:
OurHashBiMapStorageStrategy, which will eliminate this shortcoming.
16
Task
Java Collections,
level 6,
lesson 15
Locked
Shortener (12)
It's not all that uncommon to need to create a Map that works in two directions (from key to
value, and from value to key). Implementations of such collections
already exist in various third-party libraries. One such is guava from
Google.
12.1. Download and connect the guava library, version 19.0.
16
Task
Java Collections,
level 6,
lesson 15
Locked
Shortener (13)
Consider another implementation of BiMap — this time from Apache Commons Collections.
13.1. Download and connect Apache Commons Collections 4.0.
13.2. Implement a DualHashBidiMapStorageStrategy. It must:
13.2.1. support the StorageStrategy interface.
32
Task
Java Collections,
level 6,
lesson 15
Locked
Shortener (14)
We've tested our strategies many times using the Solution class's
testStrategy() method. It's time to use JUnit to write real unit tests.
14.1. Read about unit tests.
14.2. Download and connect the JUnit 4.12 library. Figure out how to use it.
The JUnit library depends on the hamcrest-core library.
16
Task
Java Collections,
level 6,
lesson 15
Locked
Shortener (15)
We're going to write another test that verifies that using HashBiMapStorageStrategy to get the identifier for a string
can be faster than using
HashMapStorageStrategy.
15.1. Create a SpeedTest class in the tests package.
9
Task
Java Collections,
level 6,
lesson 15
Locked
Shortener (16)
Stuff you can do on your own (we won't test you on any of these items):
- Add a strategy for working with a database. Google JDBC.
- Make a web service that returns an identifier for any URL or string,
and returns the string for an identifier.
GO TO FULL VERSION