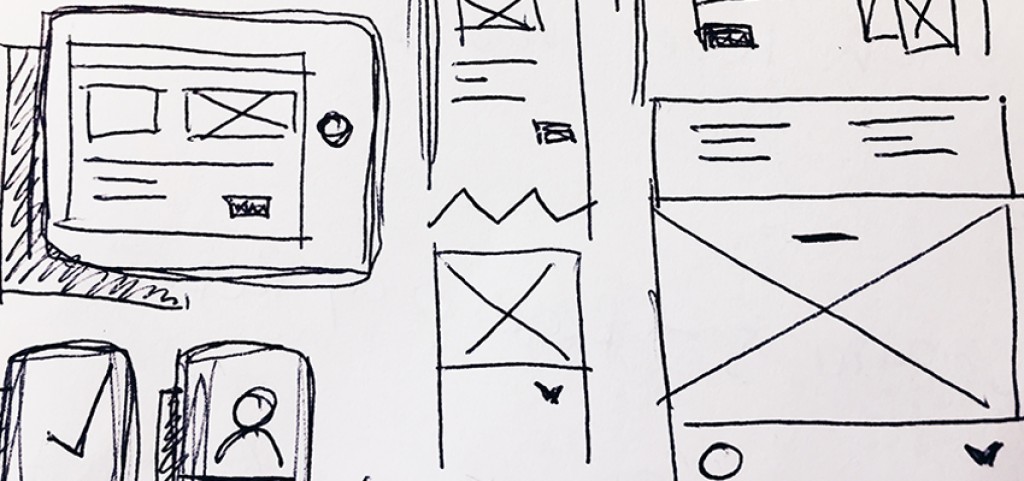
What is an Abstract Method?
An abstract method is a method that has no implementation. That is, it just has a declaration, so you know the name, the return type, and the variables it will accept. Here is an example of a basic abstract method:
public abstract int example(int a1, int a2);
When you look at this method, you can tell that it returns an integer and it accepts two integers as its argument. What you can’t tell is how this method is implemented. That’s because to implement it, you have to override it.
When creating an abstract method in java, you have to adhere to a few guidelines or your program will not compile correctly. Remember:
Java abstract methods have no implementation. That is, they should never be followed by curly braces and a body that tells how the method is to be used. It is just ended with a semicolon.
If you create an abstract method, that can only be put into an abstract class. That is, you cannot have a concrete class that has an abstract method inside of it.
i. As a side note, if you have an abstract class, it can contain constructors. It does not have to have an abstract method, however.When a concrete class extends an abstract class, it must also implement all of the abstract methods of the parent class or it cannot be concrete and must be declared abstract.
Extending Abstract Java Classes
Let’s say that we want to write a program about basic shapes that will return perimeter and area. So we create a parent abstract class. But because every shape has its own rules, each must be calculated differently, so we write the abstract Shape class like this:
abstract class Shape {
String shapeName = " ";
Shape(String name) {
this.shapeName = name;
}
abstract double area();
abstract double perimeter();
}
Now, if we want to actually use these abstract methods, we have to extend the abstract Java parent class Shape and then instantiate the methods. So each concrete class must implement the area and perimeter abstract methods.
class Quadrilateral extends Shape
{
double length, width;
Quadrilateral(double l, double w, String name)
{
super(name);
this.length = l;
this.width = w;
}
@Override
public double perimeter()
{
return ((2*length)+(2*width));
}
@Override
public double area()
{
return (length*width);
}
}
Implementing the Quadrilateral class would then look like this
class Main
{
public static void main (String[] args)
{
// creating a Quadrilateral object using Shape as reference
Shape rectangle = new Quadrilateral(3,4, "Rectangle");
System.out.println("Area of rectangle is " + rectangle.area());
System.out.println("Perimeter of rectangle is " + rectangle.perimeter());
}
}
The output from the console then looks like this:
Area of rectangle is 12.0
Perimeter of rectangle is 14.0
Note that the class Quadrilateral does not have to instantiate Shape(String name) constructor from the parent class Shape. That’s because it is not an abstract method. However, if you only implemented an area or perimeter in a class, the new class would have to be abstract because it did not include both.
You can also use abstract methods in Interfaces.
Abstract Java Methods with Interfaces
Let’s quickly review what an interface is and how it differs from an abstract class. In an interface, any variables declared in an interface are public, static, and final. Abstract classes on the other hand, only have non-final variables. Everything in an interface is public by default. An abstract class can have private, protected, public, etc. Finally, a class doesn’t extend an interface, it implements it. Previous to JDK 8, an interface could not have anything in it but abstract methods. Now, an interface can have default and static methods. Because of this, best practices have moved away from using abstract methods as extendable templates and focused on Interfaces and implementing them. So if you were to create Shape as an Interface and then implement it as Quadrilateral, what would it look like? First, you would have to do away with Shape(String name) constructor. It would look like this with just the two abstract methods:
interface Shape {
abstract double area();
abstract double perimeter();
}
So the Quadrilateral class would then look like this:
class Quadrilateral implements Shape {
double length, width;
Quadrilateral(double l, double w) {
this.length = l;
this.width = w;
}
@Override
public double perimeter() {
return ((2*length)+(2*width));
}
@Override
public double area() {
return (length*width);
}
}
Finally, using the new Quadrilateral as it implements the Shape interface would be much the same:
class Main
{
public static void main (String[] args)
{
// creating a Quadrilateral object using Shape as reference
Shape rectangle = new Quadrilateral(3,4);
System.out.println("Area of rectangle is " + rectangle.area());
System.out.println("Perimeter of rectangle is " + rectangle.perimeter());
}
}
And the console print out would look like this:
Area of rectangle is 12.0
Perimeter of rectangle is 14.0
If you’d like to explore more about the differences between interfaces and abstract classes, you can find more information here.
But Why Use Abstract Java Methods?
There are many reasons why abstract methods in Java are used and why you should get comfortable with using them. Here are three quick reasons why you should use them when it’s appropriate.Avoid duplication of efforts – Look back at our example coding; imagine that you and your team needed to create classes for shapes other than a rectangle. How many different ways are there that you could come up with to design that class? Ten? Fifteen? And that’s a simple problem. Imagine something much more complex. You and your team could come up with a hundred ways. Then you would be faced with the daunting task of weaving them together into a coherent program. This brings us to our next point: defining implementation.
Abstract Methods in Java allow the definition of use and implementation – When you use an abstract class or interface, and by design, abstract methods, you are defining how other people will interact with your interface. That lets them know what variables they should be using and what return types they can expect.
While they can override them and create concrete classes that implement your interface in unique ways, you still set in stone the core use for your code. If someone wants to implement Shape, then they have to override or implement both perimeter and area.- Readability and Debugging – Having abstract methods will enhance the readability of your code. When you write a class that implements an interface, you know what to look for. You know that every abstract method in the interface will be in the implementation, and that makes it easier to read and to track down any bugs. Abstract methods are just the beginning of learning how to properly use polymorphism in Java and other object-oriented languages. When you begin to understand and use them, an entirely new chapter of your coding journey will begin.
GO TO FULL VERSION