A lot of beginners jumble up the concept of Arrays.asList() method with the data structure ArrayList. Even though they might look and sound similar, these two are totally different when it comes to implementation.
In this post, we’ll cover the basic use of Arrays.asList() method and will bust some prevailing confusions related to it.
![Arrays.asList() Method in Java - 1]()
Why is Arrays.asList() used?
If you have an Array that you need to turn into a list then java.util.Arrays provides a wrapper Arrays.asList() to serve this purpose. In simple words, this method takes an array as a parameter and returns a list. Major portions of the Java platform API were developed before the collections framework was introduced. So occasionally, you might need to translate between traditional arrays and the more modern collections. This function serves as a link between Collections and Array based API’s.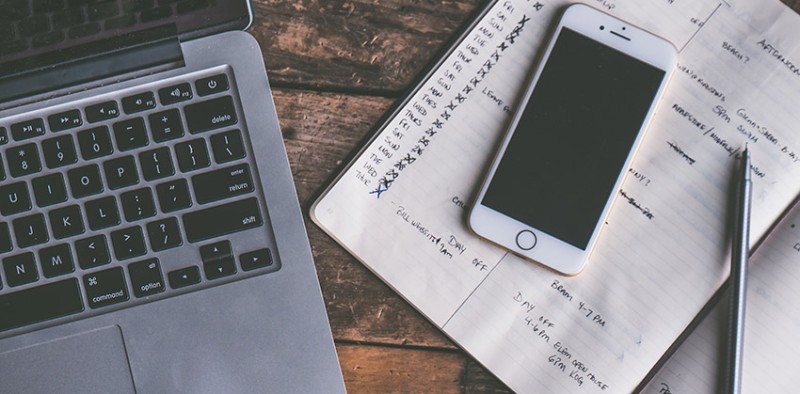
Example
Have a look at the following example:
import java.util.Arrays;
import java.util.HashSet;
import java.util.List;
public class ArraysAsListDemo {
public static void main(String[] args) {
String[] teamMembers = {"Amanda", "Loren", "Keith"};
// using aslist() method
List teamList = Arrays.asList(teamMembers);
System.out.println("List : " + teamList);
HashSet teamHashSet = new HashSet<>(Arrays.asList(teamMembers));
System.out.println("HashSet : " + teamHashSet);
}
}
Output:
List : [Amanda, Loren, Keith]
HashSet : [Keith, Loren, Amanda] // HashSet does not maintain order
How Arrays.asList() and ArrayList are different?
When you call the Arrays.asList() method on an array, the returned object is not an ArrayList (A resizable array implementation of List interface). It is a view object with get() and set() methods that access the underlying array. All methods that would change the size of the array such as the add() or remove() of the associated iterator throw an UnsupportedOperationException. The reason java program compiles successfully but gives a Runtime Exception is that, apparently, a “List” is returned as result of Arrays.asList(). Where all the addition/deletion operations are permissible. But since, the underlying data structure is a non-resizable “array”, hence an exception is thrown at the run time. Here’s a snippet showing how it looks like:
import java.util.Arrays;
import java.util.List;
public class ArraysAsListDemo {
public static void main(String[] args) {
Integer[] diceRoll = new Integer[6];
//using aslist() method
List diceRollList = Arrays.asList(diceRoll);
System.out.println(diceRollList);
// using getters and setters to randomly access the list
diceRollList.set(5, 6);
diceRollList.set(0, 1);
System.out.println(diceRollList.get(5));
System.out.println(diceRollList.get(1));
System.out.println(diceRollList);
diceRollList.add(7); // Add a new Integer to the list
}
}
Output:
[null, null, null, null, null, null]
6
null
[1, null, null, null, null, 6]
Exception in thread "main" java.lang.UnsupportedOperationException
at java.util.AbstractList.add(AbstractList.java:148)
at java.util.AbstractList.add(AbstractList.java:108)
at ArraysAsListDemo.main(ArraysAsListDemo.java:20)
Examples for using asList() method
As of Java SE 5.0, the asList() method is declared to have a variable number of arguments. Instead of passing an array, you can also pass individual elements. For example:
import java.util.Arrays;
import java.util.List;
public class ArraysAsListDemo {
public static void main(String[] args) {
List seasons = Arrays.asList("winter", "summer", "spring", "fall");
List odds = Arrays.asList(1, 3, 5, 7, 9);
System.out.println(seasons);
System.out.println(odds);
}
}
Output:
[winter, summer, spring, fall]
[1, 3, 5, 7, 9]
GO TO FULL VERSION