Why do we need to check if a file “exists?”
While dealing with file operations (read/write/create/delete/update etc), many newbies can wonder why do we even need to check if a file exists? The appropriate response to this would be, in order to avoid NoSuchFileException, it’s always a safer way to access a file. Consequently, you need to check if a file exists prior to accessing it to avoid any runtime exceptions.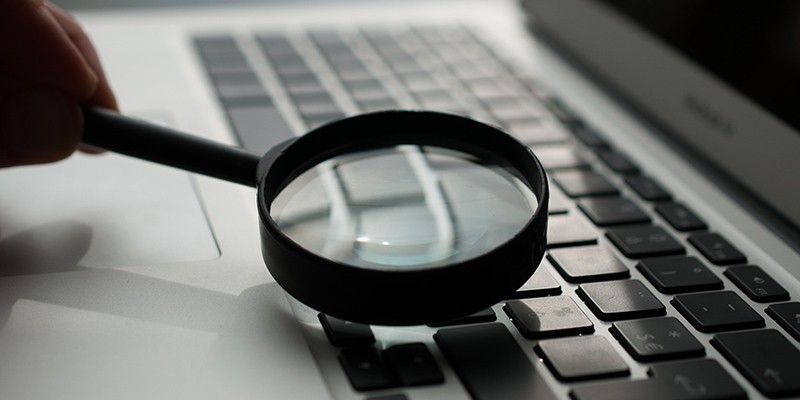
How to check using the file.exists() method?
Java provides a simple boolean method, file.exists() that doesn’t require any parameters to check the relevant file on a given path. When checking for the existence of a file, keep 3 scenarios under consideration.- The file is found.
- The file is not found.
- The file status is unknown if permissions not granted (due to security reasons).
Example
Let’s have a look at a simple code example to see the implementation.
package com.java.exists;
import java.io.File;
public class ExistsMethodInJava {
public static void main(String[] args) {
String filePath = "C:\\Users\\Lubaina\\Documents\\myNewTestFile.txt";
File file = new File(filePath);
// check if the file exists at the file path
System.out.println("Does File exists at \"" + filePath + "\"?\t" + file.exists());
filePath = "C:\\Users\\Lubaina\\Documents\\myOtherTestFile.txt";
File nextFile = new File(filePath);
// check if the file exists at the file path
System.out.println("Does File exists at \"" + filePath + "\"?\t" + nextFile.exists());
}
}
Output
Does File exists at "C:\Users\Lubaina\Documents\myNewTestFile.txt"? true
Does File exists at "C:\Users\Lubaina\Documents\myOtherTestFile.txt"? false
Kindly note that file.exists() method also works for “directory” paths. If you check a valid directory path with this method, it will return true or else false otherwise. For a better understanding, you can have a look at the following block of code.
package com.java.exists;
import java.io.File;
public class CheckFileExists {
// check if the "file" resource exists and not "directory"
public static boolean checkFileExists(File file) {
return file.exists() && !file.isDirectory();
}
public static void main(String[] args) {
String directoryPath = "C:\\Users\\Lubaina\\Documents\\javaContent";
File direcotry = new File(directoryPath);
// check if the directory exists at the dir path
if (direcotry.exists()) {
System.out.println("Direcotry at \"" + directoryPath + "\" exists.\n");
} else {
System.out.println("Direcotry at \"" + directoryPath + "\" does not exist.\n");
}
// check if the resource present at the path is a "file" not "directory"
boolean check = checkFileExists(direcotry);
System.out.println("Is the resource \"" + direcotry + "\" a File? " + check);
String filePath = "C:\\Users\\Lubaina\\Documents\\myNewTestFile.txt";
File file = new File(filePath);
check = checkFileExists(file);
System.out.println("Is the resource \"" + file + "\" a File? " + check);
}
}
Output
Directory at "C:\Users\Lubaina\Documents\javaContent" exists.
Is the resource "C:\Users\Lubaina\Documents\javaContent" a File? false
Is the resource "C:\Users\Lubaina\Documents\myNewTestFile.txt" a File? true
As you can see from the output, the Directory named “javaContent” has been validated by exists() method. So if you specifically want to check that a file is not a directory, you can use the boolean method isDirectory() provided by the File class in Java.
Conclusion
By the end of this post, you must be familiar with how to check if a file exists in Java. You can write your own programs to test and understand this functionality. Once you’re comfortable with it, you can explore other ways of checking the presence of a File (eg, using symbolic links or nio class) too. Good luck and happy coding! :)More reading: |
---|
GO TO FULL VERSION