What is the absolute value function in Mathematics?
In mathematics, the absolute value of a number is equal to the positive value of the number passed.The absolute value function ignores the sign and returns the value without it. For example, the absolute of +5 is 5. Whereas, the absolute of -5 is also 5.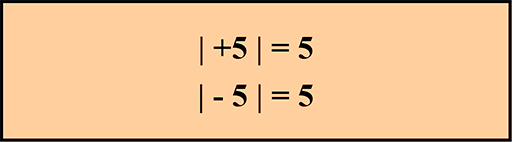
What is Math.abs() method() in Java?
Method Header
public static dataType abs(dataType parameter)
Allowed DataTypes
The abs() method of Java is overloaded for various data types. The allowed types are as under.int
float
double
long
Example 1
public class DriverClass {
public static void main(String args[]) {
int number = +5;
// Print the original number
System.out.println("Original Number = " + number);
// Printing the absolute value
// Calling the Math.abs() method
System.out.println("Absolute Number = " + "Math.abs( " + number + " ) = " + Math.abs(number));
number = -5;
// Print the original number
System.out.println("Original Number = " + number);
// Printing the absolute value
// Calling the Math.abs() method
System.out.println("Absolute Number = " + "Math.abs( " + number + " ) = " + Math.abs(number));
}
}
Output
Original Number = 5
Absolute Number = Math.abs( 5 ) = 5
Original Number = -5
Absolute Number = Math.abs( -5 ) = 5
Explanation
In the code snippet above, we have taken two numbers. The first number is a positive integer i.e. +5. The second number is a negative integer i.e. -5. We pass both the numbers to the Math.abs(number) method. The method returns 5 for both the inputs ignoring their respective signs.Example 2
public class DriverClass {
public static void main(String args[]) {
int number = -0;
System.out.println("Original Number = " + number);
System.out.println("Math.abs( " + number + " ) = " + Math.abs(number) + "\n");
long number1 = -4499990;
System.out.println("Original Number = " + number1);
System.out.println("Math.abs( " + number1 + " ) = " + Math.abs(number1) + "\n");
float number2 = -92.45f;
System.out.println("Original Number = " + number2);
System.out.println("Math.abs( " + number2 + " ) = " + Math.abs(number2) + "\n");
double number3 = -63.7777777777;
System.out.println("Original Number = " + number3);
System.out.println("Math.abs( " + number3 + " ) = " + Math.abs(number3) + "\n");
}
}
Output
Original Number = 0
Math.abs( 0 ) = 0
Original Number = -4499990
Math.abs( -4499990 ) = 4499990
Original Number = -92.45
Math.abs( -92.45 ) = 92.45
Original Number = -63.7777777777
Math.abs( -63.7777777777 ) = 63.7777777777
Explanation
In the code above, we have taken double, long and float values in addition to integer as inputs for the Math.abs() method. We have passed all the respectives values to the Math.abs() method one by one and displayed the results on the console.Boundary Cases
Here are some exceptional cases that you need to take care of while using Math.abs() method.For int and long data types
If the argument is positive zero or negative zero, the result is positive zero.Math.abs(+0) = 0
Math.abs(-0) = 0
For Integer.MIN_VALUE or Long.MIN_VALUE the output of Math.abs() is still the smallest integer or long which is negative.
Math.abs(Integer.MIN_VALUE) = -2147483648
Math.abs(Long.MIN_VALUE) = -9223372036854775808
For float and double data types
If the argument is infinite, the result is positive infinity.Math.abs(Double.NEGATIVE_INFINITY) = Infinity
If the argument is NaN, the result is NaN.
Math.abs(Double.NaN) = NaN
GO TO FULL VERSION