The JVM cannot directly run the code you write. It only knows how to execute programs written in bytecode. Bytecode is a low-level language that is close to machine code.
For Java, compilation is the translation of a (high level) program written in Java into the same program written in bytecode.
Your code is passed in a .java file to the compiler. If the compiler finds no errors in the code, then you get back a new bytecode file. This file will have the same name but a different extension: .class. If there are errors in the code, then the compiler will tell you "the program did not compile". Then you need to read each error message and fix the errors.
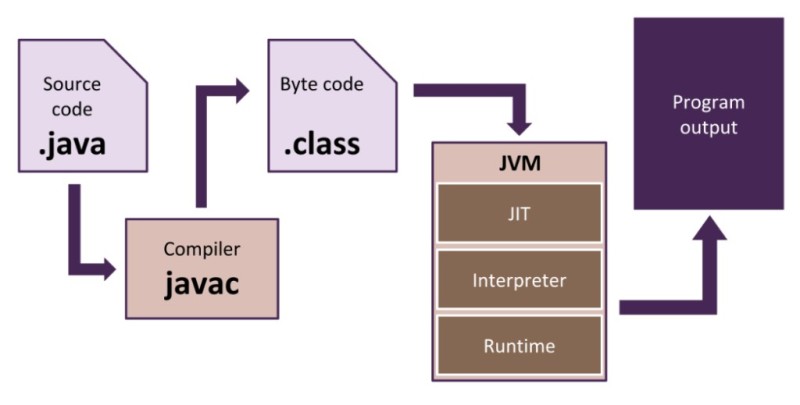
You use the javac command to invoke the compiler, which is part of the JDK (Java Development Kit). That means that if you install only the JRE (Java Runtime Environment), then you won't have the compiler! You will only have the JVM, which can only run bytecode. So we install the JDK and pass our .java file to the compiler.
For example, let's take a simple program with some console output:
class MySolution {
public static void main(String[] args) {
System.out.println("Hi, command line!");
}
}
Let's save this code to the file D:/temp/MySolution.java.
Let's compile our code using the command
D:\temp>javac MySolution.java
If there are no compilation errors in the code, a MySolution.class file will appear in the temp folder. What's more, the MySolution.java file containing your code doesn't go anywhere. It remains right where it was. But now MySolution.class contains bytecode and is ready to be run directly by the JVM.
Our example is as simple as possible, but even the largest and most complex projects use the javac command. So it will be very useful for you to know how it works.
You can compile more than one class at a time. For example, suppose there is another file Cat.java in the temp folder:
D:\temp>javac MySolution.java Cat.java
or this:
D:\temp>javac *.java
Once compiled, the .class files can be packaged in a jar file, which can be conveniently moved to different computers and then run on them. Here's an example of how to create a jar file:
D:\temp>jar cvfe myjar.jar MySolution MySolution.class Cat.class
where:
jar is the command for creating .jar files, included in the JDK
cvfe are command line options with the following meanings:
c – create a new jar file
v – display verbose information
f – indicates that we want the tool's output to be put into a file (myjar.jar)
e – indicates the entry point (MySolution), i.e. the class that contains the main method
MySolution.java Cat.class – space-separated names of the files that will be packed into the jar file.
Here's how we run the resulting jar file:
D:\temp>java -jar myjar.jar
For more detailed information: |
---|
The javac and jar tools have built-in help. To display it, run the following on the command line:
|