In programming, there are situations where we need to work with pairs of values, where each value has a corresponding key associated with it. That is, a pair is a container for storing a tuple of two objects.
In C++ programming language, there is a template class std::pair in the utility library. It keeps two values of the same type. These values are called the first and second element of the pair. Pairs can be used to represent various data types such as coordinates, points, colors, and so on. They are also commonly used in C++ container libraries such as std::map and std::set.
There is no built-in Pair class in the core Java Development Kit (JDK) library. However, many developers use third-party libraries or implementations of the Pair classes provided by various frameworks and libraries to work with value pairs in Java.
First of all, these are libraries such as Apache Commons and Guava, which provide their own class implementations for working with pairs and tuples of values.
Also, if you need a Pair class, you can use one of these third party libraries or create your own Pair class to suit your needs.
Here is an example of using the Pair class in Java:
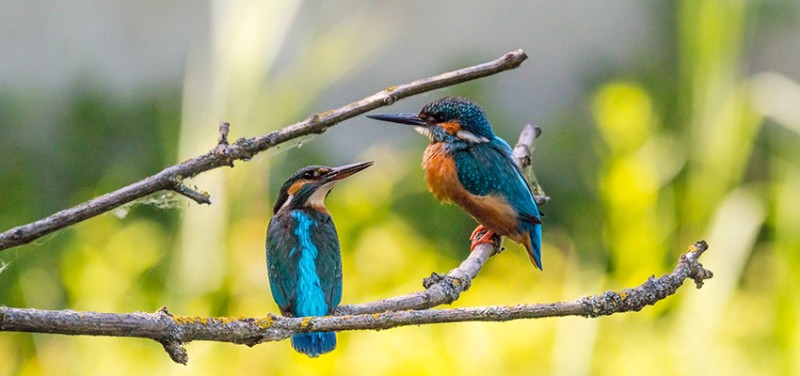
Pair Class in JavaFX
The Pair in Java is a part of the javafx.util package and is used to represent a pair of values, where one value is associated with a key (key to value relationship). Although it is not a built-in class in the standard Java library, it provides a simple and straightforward way to manage key/value pairs. The Pair class has two fields: key and value, representing the key and value associated with the pair, respectively. This makes it a handy container to hold related data together.Example of Using Pair Class
Here's an example of how to use the Java Pair class:
import javafx.util.Pair;
public class PairExample {
public static void main(String[] args) {
//key and value
Pair<String, Integer> agePair = new Pair<>("Alice", 25);
System.out.println("Name: " + agePair.getKey());//get key
System.out.println("Age: " + agePair.getValue());
}
}
Output:
Name: Alice
Age: 25
In this example, we create a Pair instance named agePair containing a name (key) and an age (value). We then retrieve and print the name and age using the getKey() and getValue() methods.
Let's consider another example where we use pairs to represent and display country names along with their corresponding capitals:
import javafx.util.Pair;
public class CountryCapitalExample {
public static void main(String[] args) {
Pair<String, String> countryCapitalPair = new Pair<>("France", "Paris");
System.out.println("Country: " + countryCapitalPair.getKey());//get key
System.out.println("Capital: " + countryCapitalPair.getValue());
}
}
Output:
Country: France
Capital: Paris
Pairs in Apache Commons Java
Apache Commons Lang is a widely used library that provides a rich set of utility classes for various common programming tasks in Java. Among its many features, Apache Commons Lang includes a Pair class that allows developers to work with pairs of values in a convenient way. This can be particularly useful when you need to return two values from a method or store related data together. Here's an example of how to use Pair in Apache Commons:
import org.apache.commons.lang3.tuple.Pair;
public class PairExample {
public static void main(String[] args) {
// Creating a Pair of values
Pair<String, Integer> nameAndAge = Pair.of("John", 30);
// Accessing the values
String name = nameAndAge.getLeft();
int age = nameAndAge.getRight();
// Printing the values
System.out.println("Name: " + name);
System.out.println("Age: " + age);
}
}
In this example, we import the Pair class from Apache Commons Lang and create a Pair of a String (name) and an Integer (age). We can then easily access and manipulate these values using the getLeft() and getRight() methods. Apache Commons Lang provides a convenient way to work with pairs of values, making your code more readable and maintainable.
Pair class in Guava library
In the Guava library, you can work with pairs using the Pair class provided by the com.google.common.collect package. Guava's Pair is similar to Apache Commons Lang's Pair and allows you to represent a simple pair of two values. Here's an example of how to use Pair in Guava:
import com.google.common.collect.*;
public class GuavaPairExample {
public static void main(String[] args) {
// Creating a Pair of values
Pair<String, Integer> nameAndAge = Pair.of("John", 30);
// Accessing the values
String name = nameAndAge.getFirst();
int age = nameAndAge.getSecond();
// Printing the values
System.out.println("Name: " + name);
System.out.println("Age: " + age);
}
}
In this example, we create a Pair consisting of a String (name) and an Integer (age) using .of(). We then access the values using getFirst() and getSecond() methods.
Here is the output of the program:
Name: John
Age: 30
In this program we import the necessary classes from Guava. Then we create a Pair named nameAndAge with the values "John" and 30, retrieve the values using getFirst() and getSecond(). Finally, we print the values to the console.
Guava's Pair is a straightforward way to work with pairs of values, making your code more expressive and readable when you need to handle pairs of related data.
Your own Pair class in Java
As we said before the pair library in C++ provides a convenient way to store two values together. The pair class has two elements: first and second. Both elements can be of any type, including template classes. Here is a simple analogue of the Pair class in Java:
public class Pair<T, U> {
private T first;
private U second;
public Pair(T first, U second) {
this.first = first;
this.second = second;
}
public T getFirst() {
return first;
}
public void setFirst(T first) {
this.first = first;
}
public U getSecond() {
return second;
}
public void setSecond(U second) {
this.second = second;
}
@Override
public String toString() {
return "Pair{" +
"first=" + first +
", second=" + second +
'}';
}
}
This class has two constructors: one that takes two parameters, and one that takes no parameters. If the constructor that takes no parameters is used, then both elements of the pair are initialized to null.
Pair<Integer, String> pair1 = new Pair<>(1, "one");
Pair<Double, Character> pair2 = new Pair<>(3.14, 'pi');
System.out.println("pair1.first = " + pair1.getFirst());
System.out.println("pair1.second = " + pair1.getSecond());
System.out.println("pair2.first = " + pair2.getFirst());
System.out.println("pair2.second = " + pair2.getSecond());
This program outputs the following result:
pair1.first = 1
pair1.second = one
pair2.first = 3.14
pair2.second = pi
This analogue of the pair class is fairly simple, but it can be used to store two values of any type.
GO TO FULL VERSION