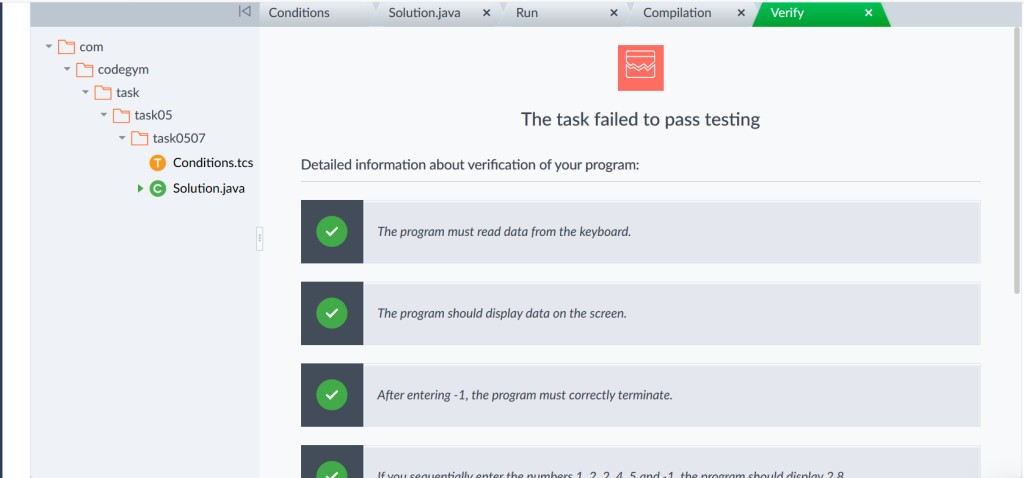
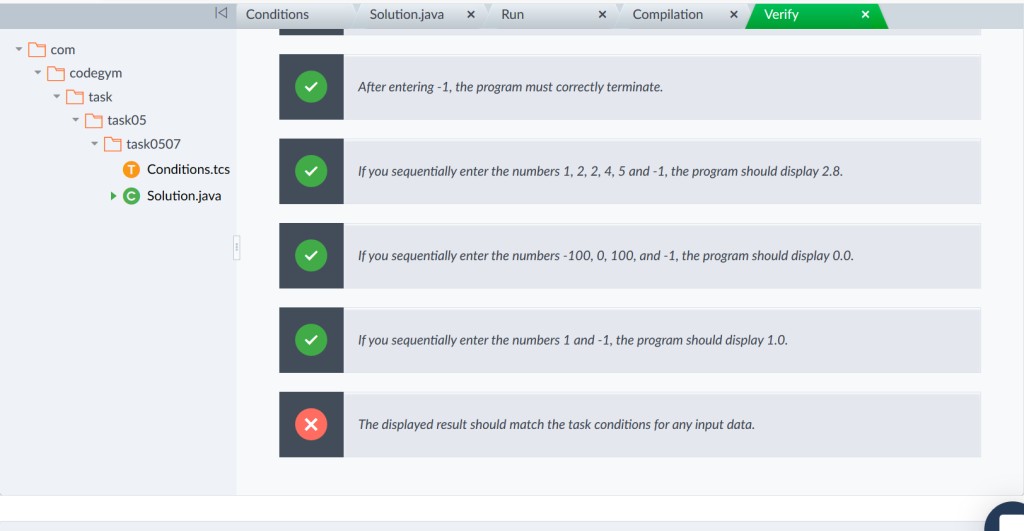
package com.codegym.task.task05.task0507;
/*
Arithmetic mean
*/
import java.io.*;
public class Solution {
public static void main(String[] args) throws Exception {
//write your code here
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
float mean = 0;
float sum = 0;
float counter = 0;
while (true){
String input = reader.readLine();
float number = Float.parseFloat(input);
if (number==-1){
break;
}
else if (number<0 || number>0){
sum = sum+number;
counter++;
}
}
mean = sum/counter;
System.out.println(mean);
}
}