package com.codegym.task.task06.task0621;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
/*
Cat relations
*/
public class Solution {
//public Cat a=null;
public static void main(String[] args) throws IOException {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
String gfatherName = reader.readLine();
Cat catgFather = new Cat(gfatherName);
String gmotherName = reader.readLine();
Cat catgMother = new Cat(gmotherName);
String fatherName = reader.readLine();
Cat catFather = new Cat(fatherName,catgFather,null);
String motherName = reader.readLine();
Cat catMother = new Cat(motherName,null,catgMother);
String sonName = reader.readLine();
Cat catSon = new Cat(sonName,catFather, catMother);
//order of arguments not matter because last two are of same type;)wrong mather order of creation of objs
String daughterName = reader.readLine();
Cat catDaughter = new Cat(daughterName, catFather ,catMother);
System.out.println(catgFather);
System.out.println(catgMother);
System.out.println(catFather);
System.out.println(catMother);
System.out.println(catSon);
System.out.println(catDaughter);
}
public static class Cat {
private String name;
private Cat parent;
private Cat parenti;
Cat(String name) {
this.name = name;
}
Cat(String name, Cat parent,Cat parenti){
this.name = name;
this.parent = parent;
this.parenti=parenti;
}
@Override
public String toString() {
if (parent != null && parenti!=null)
return "The cat's name is " + name + ", " + parenti.name +" is the mother" + parent.name + " is the father";
else if(parent!=null && parenti==null)
return "The cat's name is " + name + ", " +" no mother "+parent.name + " is the father";
else if (parenti!=null && parent==null)
return "The cat's name is " + name + ", " +" no father "+ parent.name + " is the mother";
else
return "The cat's name is " + name + ", no mother " + ", no father ";
}
}
}
![]()
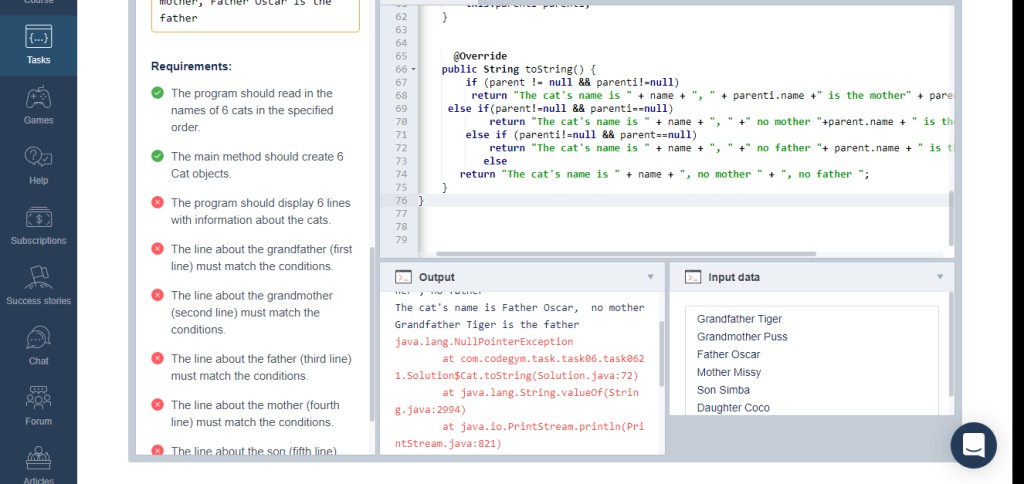